用c语言实现:图书表抽象成一个线性表,每本图书(包括ISBN、书名、定价)作为线性表中的一个元素,该线性表采用链式存储结构,对该线性表进行初始化、创建、输出、求长度、插入和删除基本操作,请编写代码实现。
时间: 2024-10-13 08:17:06 浏览: 39
在C语言中,我们可以使用链表数据结构来模拟图书表,每个链表节点包含书籍的信息。下面是一个简单的实现,包括了初始化、创建、输出、求长度、插入和删除的基本操作:
首先,我们需要定义一个链表节点结构体:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct BookNode {
char isbn[20];
char title[100];
float price;
struct BookNode* next;
} BookNode;
```
然后是链表的操作函数:
```c
// 初始化链表
void initList(BookNode** head) {
*head = NULL;
}
// 创建链表并插入元素
void createList(BookNode** head, char isbn[], char title[], float price) {
BookNode* newNode = (BookNode*) malloc(sizeof(BookNode));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
exit(1);
}
strcpy(newNode->isbn, isbn);
strncpy(newNode->title, title, sizeof(newNode->title)-1); // 防止溢出
newNode->price = price;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
BookNode* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 输出链表
void displayList(BookNode* head) {
BookNode* temp = head;
while (temp != NULL) {
printf("ISBN: %s, Title: %s, Price: %.2f\n", temp->isbn, temp->title, temp->price);
temp = temp->next;
}
}
// 求链表长度
size_t listLength(BookNode* head) {
size_t length = 0;
while (head != NULL) {
length++;
head = head->next;
}
return length;
}
// 插入新元素到链表末尾
void insertAtEnd(BookNode** head, char isbn[], char title[], float price) {
createList(head, isbn, title, price);
}
// 删除指定ISBN的图书
void deleteByISBN(BookNode** head, char isbn[]) {
if (*head == NULL) return;
if ((*head)->isbn[0] == '\0') {
*head = (*head)->next;
free(*head);
return;
}
BookNode* prev = *head;
BookNode* curr = *head->next;
while (curr != NULL && strcmp(curr->isbn, isbn) != 0) {
prev = curr;
curr = curr->next;
}
if (curr == NULL) return;
if (prev == *head) {
*head = curr->next;
} else {
prev->next = curr->next;
}
free(curr);
}
```
请注意,上述代码假设输入的字符串不会超过最大长度,并且删除操作只针对首部匹配的节点。在实际应用中,可能需要更复杂的功能,如错误检查和边界条件处理。
阅读全文
相关推荐
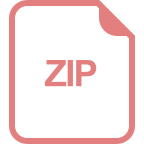
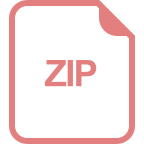















