写一个几何体类给出计算面积的纯虚函数;,三角形类继承几何体类重写计算面积的函数,矩形继承几何体类计算面积的函数 写一个几何体为底的柱体类,给出计算体积的函数 写main测试自己的类和函数 理解开闭原则 2. 使用c语言实现1中描述的功能
时间: 2024-10-15 18:17:40 浏览: 35
在C++中,我们可以创建一个名为`Geometry`的抽象基类,定义一个纯虚函数`calculateArea()`,表示所有几何体都应有自己的面积计算方法。然后我们分别创建`Triangle`和`Rectangle`作为`Geometry`的派生类,并实现各自的面积计算。
```cpp
#include <iostream>
// 抽象基类 Geometry
class Geometry {
public:
virtual double calculateArea() const = 0; // 纯虚函数,用于计算面积
};
// 子类 Triangle 继承 Geometry 并重写 calculateArea()
class Triangle : public Geometry {
private:
double base;
double height;
public:
Triangle(double b, double h) : base(b), height(h) {}
double calculateArea() const override {
return 0.5 * base * height;
}
};
// 子类 Rectangle 继承 Geometry 并计算面积
class Rectangle : public Geometry {
private:
double length;
double width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double calculateArea() const override {
return length * width;
}
};
// 柱体类 Cylinder 以几何体为底,计算体积
class Cylinder {
private:
Geometry* baseGeometry;
double height;
public:
Cylinder(Geometry* geom, double h) : baseGeometry(geom), height(h) {}
double calculateVolume() const {
return M_PI * pow(*baseGeometry->calculateArea(), 2) * height;
}
};
int main() {
Triangle triangle(3, 4);
std::cout << "Triangle area: " << triangle.calculateArea() << std::endl;
Rectangle rectangle(5, 6);
std::cout << "Rectangle area: " << rectangle.calculateArea() << std::endl;
Geometry* cylinderBase = new Triangle(triangle.base, triangle.height);
Cylinder cylinder(cylinderBase, 7);
delete cylinderBase; // 注意内存管理
std::cout << "Cylinder volume with Triangle base: " << cylinder.calculateVolume() << std::endl;
return 0;
}
// 解开闭原则(Liskov替换原则):新创建的Cylinder类可以使用任何实现了Geometry接口的对象作为基础,保证了程序的灵活性和扩展性。
//
阅读全文
相关推荐
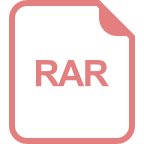
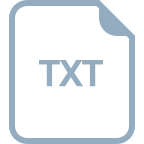
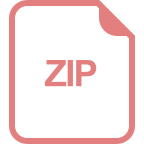















