基于python链表实现个人资产管理系统
时间: 2024-01-04 12:44:29 浏览: 27
下面是一个简单的基于Python链表的个人资产管理系统:
```python
class Asset:
def __init__(self, name, value):
self.name = name
self.value = value
self.next = None
class AssetList:
def __init__(self):
self.head = None
def add_asset(self, name, value):
new_asset = Asset(name, value)
if not self.head:
self.head = new_asset
else:
current = self.head
while current.next:
current = current.next
current.next = new_asset
def remove_asset(self, name):
if not self.head:
return "Asset list is empty"
if self.head.name == name:
self.head = self.head.next
return f"{name} removed from asset list"
current = self.head
while current.next:
if current.next.name == name:
current.next = current.next.next
return f"{name} removed from asset list"
current = current.next
return f"{name} not found in asset list"
def total_value(self):
if not self.head:
return 0
total = 0
current = self.head
while current:
total += current.value
current = current.next
return total
def print_assets(self):
if not self.head:
print("Asset list is empty")
else:
current = self.head
while current:
print(f"{current.name}: ${current.value}")
current = current.next
```
上面的代码定义了两个类,一个是`Asset`,表示每一个资产,包括资产名称和价值;另一个是`AssetList`,表示资产列表。`AssetList`中有添加、删除、计算总价值和打印资产列表的方法。这些方法都是基于链表实现的。
下面是一个使用示例:
```python
asset_list = AssetList()
asset_list.add_asset("Savings", 10000)
asset_list.add_asset("Investment", 50000)
asset_list.add_asset("Real Estate", 200000)
asset_list.print_assets()
# Output:
# Savings: $10000
# Investment: $50000
# Real Estate: $200000
print(f"Total value: ${asset_list.total_value()}")
# Output: Total value: $260000
asset_list.remove_asset("Investment")
asset_list.print_assets()
# Output:
# Savings: $10000
# Real Estate: $200000
print(f"Total value: ${asset_list.total_value()}")
# Output: Total value: $210000
```
在上面的示例中,我们首先创建了一个资产列表`asset_list`,然后添加了三个资产,分别是储蓄、投资和房地产。接着打印了资产列表和总价值。然后,我们移除了投资这个资产,再次打印资产列表和总价值。可以看到,投资这个资产已经被成功移除了。
相关推荐
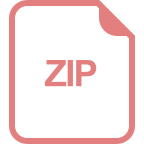
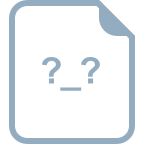













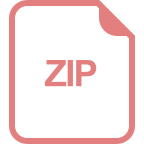