aes java加密
时间: 2023-08-29 19:10:51 浏览: 92
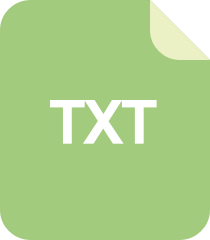
java处理常规加密解密
AES(Advanced Encryption Standard)是一种常用的对称加密算法,可以用数据的加密和解密。在Java中,你可以使用Java加密扩展(Java Cryptography Extension,JCE)中的AES算法来进行加密。
下面是一个使用AES算法进行加密的Java代码示例:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.security.SecureRandom;
import java.util.Base64;
public class AESExample {
public static void main(String[] args) {
try {
String plaintext = "Hello, world!";
String encryptionKey = "ThisIsASecretKey";
// 生成AES密钥
SecretKey secretKey = generateKey(encryptionKey);
// 加密
String encryptedText = encrypt(plaintext, secretKey);
System.out.println("Encrypted Text: " + encryptedText);
// 解密
String decryptedText = decrypt(encryptedText, secretKey);
System.out.println("Decrypted Text: " + decryptedText);
} catch (Exception e) {
e.printStackTrace();
}
}
public static SecretKey generateKey(String encryptionKey) throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
SecureRandom secureRandom = new SecureRandom(encryptionKey.getBytes());
keyGenerator.init(128, secureRandom);
return keyGenerator.generateKey();
}
public static String encrypt(String plaintext, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(plaintext.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String encryptedText, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] encryptedBytes = Base64.getDecoder().decode(encryptedText);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return new String(decryptedBytes);
}
}
```
在上面的示例中,我们使用了128位的AES密钥,并对字符串"Hello, world!"进行加密和解密。加密后的结果以Base64编码的形式打印出来。
请注意,这个示例只是为了演示AES加密算法的使用,实际应用中需要注意密钥的安全存储和传输等问题。
阅读全文
相关推荐
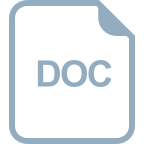
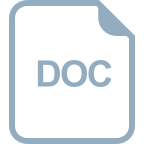
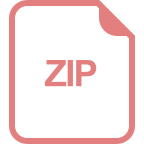
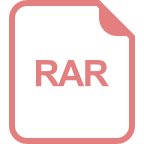
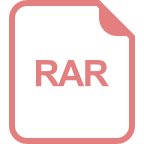
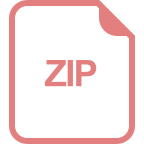
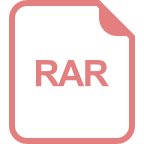
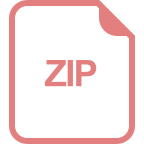
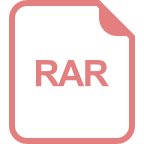
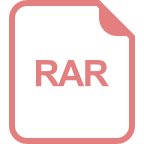
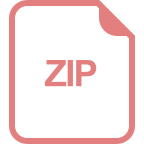
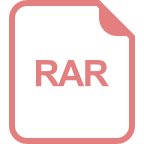
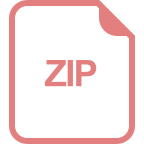
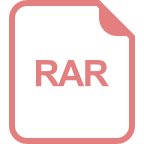
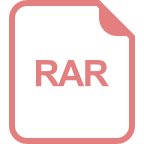
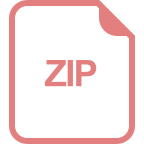
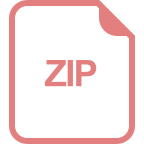
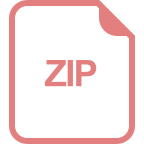