该程序打开文件“test.txt”,用lseek将读写指针定位到10处,并从此处读取10个字节(如果有的话)显示到屏幕上。最后用lseek(fd, 0, SEEK_END)计算文件长度。
时间: 2024-03-14 20:47:22 浏览: 19
好的,以下是实现该功能的示例代码:
```c++
#include <iostream>
#include <unistd.h>
#include <fcntl.h>
using namespace std;
int main() {
int fd = open("test.txt", O_RDONLY);
if (fd == -1) {
perror("open");
return 1;
}
int offset = lseek(fd, 10, SEEK_SET);
if (offset == -1) {
perror("lseek");
return 1;
}
char buf[11] = {0};
int n = read(fd, buf, 10);
if (n == -1) {
perror("read");
return 1;
}
cout << "读取到的内容为:" << buf << endl;
offset = lseek(fd, 0, SEEK_END);
if (offset == -1) {
perror("lseek");
return 1;
}
cout << "文件长度为:" << offset << endl;
close(fd);
return 0;
}
```
在上面的代码中,我们首先通过 `open` 函数打开了名为 `test.txt` 的文件,并获取到了该文件的文件描述符 `fd`。接着,我们调用 `lseek` 函数将读写指针定位到了文件的第 10 个字节,然后调用 `read` 函数读取了接下来的 10 个字节到了缓冲区 `buf` 中,并将其输出到了屏幕上。最后,我们再次调用 `lseek` 函数将读写指针定位到了文件的结尾,并调用 `close` 函数关闭了文件。
需要注意的是,上面的代码中使用了一些与操作系统相关的系统调用函数,如 `open`、`lseek`、`read` 和 `close` 等,因此在运行该程序时,需要在编译选项中加上 `-std=c++11 -lstdc++fs`,并在链接选项中加上 `-lc`。
相关推荐
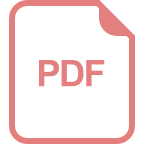
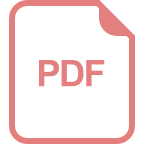














