def mhsa_block(input_layer, input_channel): # W, H = 25, 25 W, H = int(input_layer.shape[1]), int(input_layer.shape[2]) # From 2-D to Sequence: WxHxd -> W*Hxd (e.g., 25x25x512 -> 1x625x512) conv = Reshape((1, W*H, input_channel))(input_layer) # Position Encoding: 1x625x512 -> 1x625x512 pos_encoding = Conv2D(input_channel, 1, activation='relu', padding='same', kernel_initializer='he_normal')(conv) # Element-wise Sum: 1x625x512 conv = Add()([conv, pos_encoding]) # Query: Conv1x1 --> 1x625x512 conv_q = Conv2D(input_channel, 1, activation='relu', padding='same', kernel_initializer='he_normal')(conv) # Key: Conv1x1 --> 1x625x512 conv_k = Conv2D(input_channel, 1, activation='relu', padding='same', kernel_initializer='he_normal')(conv) # Value: Conv1x1 --> 1x625x512 conv_v = Conv2D(input_channel, 1, activation='relu', padding='same', kernel_initializer='he_normal')(conv) # Transposed Key: 1x512x612 conv_k = Permute(dims=(1, 3, 2))(conv_k) # Content-content: Query * Key_T --> 1x625x625 conv = Dot(axes=(3,2))([conv_q, conv_k]) conv = Reshape((1, W*H, W*H))(conv) # Softmax --> 1x625x625 conv = Softmax()(conv) # Output: Dot(1x625x625, 1x625x512) --> 1x625x512 conv = Dot(axes=(3,2))([conv, conv_v]) # From Sequence to 2-D conv = Reshape((W, H, input_channel))(conv) return conv 定义后如何调用
时间: 2024-01-14 09:03:28 浏览: 25
可以通过以下方式调用该函数:
```python
input_layer = Input(shape=(25, 25, 512))
output_layer = mhsa_block(input_layer, 512)
model = Model(inputs=input_layer, outputs=output_layer)
```
其中 `Input(shape=(25, 25, 512))` 用于定义输入特征图的形状,而 `mhsa_block(input_layer, 512)` 则是将输入特征图通过 MHSA 模块处理得到输出特征图。最后通过 `Model` 函数将输入和输出包装成一个模型,以便进行训练或者预测。
相关问题
在 '__init__.py' 中找不到引用 'MHSA'
在 '__init__.py' 中找不到引用 'MHSA' 可能是因为 'MHSA' 这个引用没有被正确导入或者没有在 '__init__.py' 文件中注册。请检查一下代码,确保 'MHSA' 这个引用被正确导入并在 '__init__.py' 文件中进行了注册。如果仍然找不到引用,可能需要进一步检查代码逻辑或者查看相关文档以获取更多信息。
#### 引用[.reference_title]
- *1* *2* *3* [改进YOLOv5 | 在 C3 模块中添加【SE】【CBAM】【 ECA 】【CA】注意力机制 | 附详细结构图](https://blog.csdn.net/weixin_43694096/article/details/130733990)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
翻译:分割:将Q, K, V向量(x''∈ℝ(H×W)×C)切片为h个低阶嵌入{x_1,x_2,∙∙∙,x_h }, 其中每个单维子空间xi∈ℝh×(H×W)×(C/h)表示MHSA中的一个头;
Segmentation: Slice the Q, K, and V vectors (x'' ∈ ℝ(H×W)×C) into h low-order embeddings {x1, x2, ..., xh}, where each single subspace xi ∈ ℝh×(H×W)×(C/h) represents a head in MHSA.
相关推荐
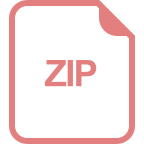
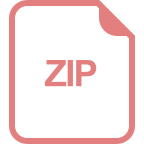
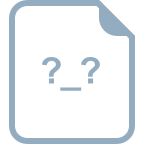












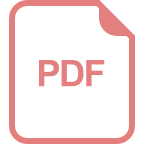
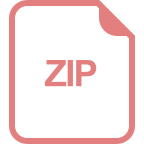