编写程序,使用进程池并行计算range对象中数值的平方,屏幕输出值同时显示当前日期与时间。已给出部分程序供参考,请编写完整的功能程序。 from multiprocessing import Po
时间: 2024-10-07 14:03:30 浏览: 32
当然可以。首先,我们需要导入必要的库,包括`multiprocessing`库用于创建进程池,`datetime`库获取当前日期和时间,以及`time`库让任务间隔运行。这里是一个简单的Python示例:
```python
import multiprocessing
import datetime
import time
def square_and_log(value, log_queue):
# 计算数值的平方
squared_value = value ** 2
# 获取当前日期和时间
current_time = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
# 将结果和时间信息放入队列
log_queue.put((current_time, squared_value))
if __name__ == '__main__':
# 创建一个进程池,最大并发数设置为4
pool = multiprocessing.Pool(processes=4)
# range对象中的数值范围
numbers = list(range(10))
# 创建一个队列用于保存每个进程的结果
result_queue = multiprocessing.Queue()
# 使用map函数将任务分发到进程池,并将结果和时间信息保存到队列
jobs = [pool.apply_async(square_and_log, args=(i, result_queue)) for i in numbers]
try:
while not all([job.ready() for job in jobs]):
# 每隔一秒检查是否有新的结果可用
time.sleep(1)
print("Checking results...")
print("\nAll jobs completed.")
while not result_queue.empty():
# 取出并打印所有的结果及其对应的时间
timestamp, squared_value = result_queue.get()
print(f"{timestamp}: {squared_value}")
except KeyboardInterrupt:
print("\nTerminating processes...")
pool.terminate()
pool.join()
finally:
pool.close()
```
这个程序会创建一个包含4个进程的工作池,然后将`range(10)`中的数字分配给这些进程,它们会计算每个数的平方并将结果连同当前时间一起存入队列。主程序会每隔1秒查询队列,直到所有进程完成。如果用户中断程序,它会终止进程。
阅读全文
相关推荐
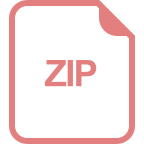
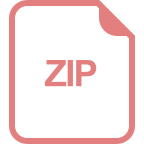
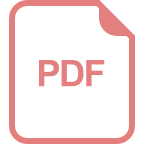
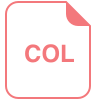
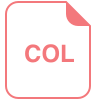
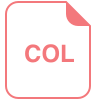
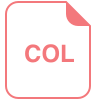
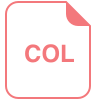
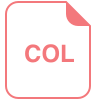
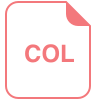
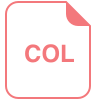
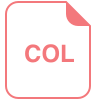
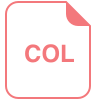
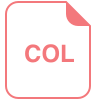
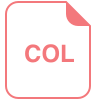
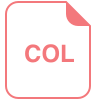
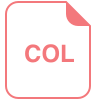
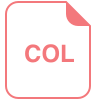
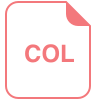