使用手写数字数据集(sklearn.datasets.load_digits())做为训练集。 1)构建随机森林(查找并学习sklearn中与随机森林相关的函数),不断改变随机森林中树的数量,并画出训练集精度随着树数量的增加(1到100)的关系曲线图
时间: 2023-06-13 18:07:06 浏览: 122
好的,我可以帮助你完成这个任务。首先,我们需要导入需要的库和数据集。请确保你已经安装了scikit-learn库。
```python
from sklearn.datasets import load_digits
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
import matplotlib.pyplot as plt
# 加载手写数字数据集
digits = load_digits()
X, y = digits.data, digits.target
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们可以使用`RandomForestClassifier`类来构建随机森林模型。我们可以通过设置`n_estimators`参数来控制树的数量。然后,我们可以使用训练集拟合模型并使用测试集评估模型的性能。
```python
# 定义树的数量范围
n_estimators_range = range(1, 101)
train_scores = []
test_scores = []
for n_estimators in n_estimators_range:
# 构建随机森林模型
model = RandomForestClassifier(n_estimators=n_estimators, random_state=42)
# 使用训练集拟合模型
model.fit(X_train, y_train)
# 计算训练集和测试集的精度
train_score = model.score(X_train, y_train)
test_score = model.score(X_test, y_test)
# 将精度分数添加到列表中
train_scores.append(train_score)
test_scores.append(test_score)
```
最后,我们可以将训练集精度和树的数量之间的关系绘制成图表。
```python
# 绘制训练集精度和树的数量之间的关系
plt.plot(n_estimators_range, train_scores, label='Train Score')
plt.xlabel('Number of Trees')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
```
完整代码如下:
```python
from sklearn.datasets import load_digits
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
import matplotlib.pyplot as plt
# 加载手写数字数据集
digits = load_digits()
X, y = digits.data, digits.target
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义树的数量范围
n_estimators_range = range(1, 101)
train_scores = []
test_scores = []
for n_estimators in n_estimators_range:
# 构建随机森林模型
model = RandomForestClassifier(n_estimators=n_estimators, random_state=42)
# 使用训练集拟合模型
model.fit(X_train, y_train)
# 计算训练集和测试集的精度
train_score = model.score(X_train, y_train)
test_score = model.score(X_test, y_test)
# 将精度分数添加到列表中
train_scores.append(train_score)
test_scores.append(test_score)
# 绘制训练集精度和树的数量之间的关系
plt.plot(n_estimators_range, train_scores, label='Train Score')
plt.xlabel('Number of Trees')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
```
运行代码后,你可以看到一个图表,它显示了训练集精度和树的数量之间的关系。随着树的数量增加,训练集精度也会增加,但是当树的数量超过一定限制时,训练集精度的增长会放缓并趋于平稳。
阅读全文
相关推荐
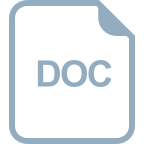
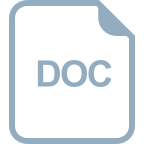
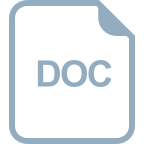















