通过Gige协议接收Gige相机图像数据代码
时间: 2023-09-17 15:11:58 浏览: 68
以下是通过GigE协议接收GigE相机图像数据的示例代码,使用了GigE Vision SDK:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <GevApi.h>
#include <SapX11Util.h>
#include <X11/Xlib.h>
#include <X11/Xutil.h>
#define MAX_NETIF 8
#define MAX_CAMERAS_PER_NETIF 32
#define MAX_CAMERAS (MAX_NETIF * MAX_CAMERAS_PER_NETIF)
#define NUM_BUF 4
#define TIMEOUT 1000
int main(int argc, char* argv[])
{
// Initialize the GigE Vision API
if (GevApiInitialize() != GEVLIB_OK)
{
printf("Error: GevApiInitialize() failed!\n");
exit(EXIT_FAILURE);
}
// Get all available network interfaces
GEV_DEVICE_INTERFACE pNetIF[MAX_NETIF];
uint32_t numInterfaces = 0;
if (GevGetInterfaces(pNetIF, MAX_NETIF, &numInterfaces) != GEVLIB_OK)
{
printf("Error: GevGetInterfaces() failed!\n");
exit(EXIT_FAILURE);
}
// Get all available cameras on the network
GEV_CAMERA pCamera[MAX_CAMERAS];
uint32_t numCameras = 0;
for (uint32_t i = 0; i < numInterfaces; i++)
{
if (GevGetCameraList(pNetIF[i].szInterfaceName, GEV_PROTOCOL_FORCEIP, pCamera + numCameras, MAX_CAMERAS_PER_NETIF, &numCameras) != GEVLIB_OK)
{
printf("Error: GevGetCameraList() failed on interface %s!\n", pNetIF[i].szInterfaceName);
exit(EXIT_FAILURE);
}
}
// Open a connection to the first camera found
GEV_CAMERA_HANDLE hCamera = NULL;
if (numCameras > 0)
{
if (GevOpenCamera(&pCamera[0], GEV_EXCLUSIVE_MODE, &hCamera) != GEVLIB_OK)
{
printf("Error: GevOpenCamera() failed!\n");
exit(EXIT_FAILURE);
}
}
else
{
printf("Error: no camera found on the network!\n");
exit(EXIT_FAILURE);
}
// Start the acquisition engine
if (GevStartImageTransfer(hCamera, NUM_BUF) != GEVLIB_OK)
{
printf("Error: GevStartImageTransfer() failed!\n");
exit(EXIT_FAILURE);
}
// Allocate buffers for incoming images
uint32_t payloadSize = 0;
if (GevGetPayloadSize(hCamera, &payloadSize) != GEVLIB_OK)
{
printf("Error: GevGetPayloadSize() failed!\n");
exit(EXIT_FAILURE);
}
void* pBuffer[NUM_BUF];
for (uint32_t i = 0; i < NUM_BUF; i++)
{
pBuffer[i] = malloc(payloadSize);
if (pBuffer[i] == NULL)
{
printf("Error: failed to allocate buffer!\n");
exit(EXIT_FAILURE);
}
if (GevRegisterImageBuffer(hCamera, pBuffer[i], payloadSize, i) != GEVLIB_OK)
{
printf("Error: GevRegisterImageBuffer() failed!\n");
exit(EXIT_FAILURE);
}
}
// Create a window to display the image
Display* pDisplay = XOpenDisplay(NULL);
if (pDisplay == NULL)
{
printf("Error: XOpenDisplay() failed!\n");
exit(EXIT_FAILURE);
}
Window window = XCreateSimpleWindow(pDisplay, DefaultRootWindow(pDisplay), 0, 0, 640, 480, 0, 0, 0);
XSelectInput(pDisplay, window, ExposureMask | KeyPressMask);
XMapWindow(pDisplay, window);
while (true)
{
// Wait for an image to be received
GEV_BUFFER_OBJECT pBufferObj = { 0 };
if (GevWaitForNextImage(hCamera, &pBufferObj, TIMEOUT) == GEVLIB_OK)
{
// Get the image data and display it
char* pData = (char*)pBuffer[pBufferObj.context];
int width = pBufferObj.w;
int height = pBufferObj.h;
XImage* pImage = XCreateImage(pDisplay, DefaultVisual(pDisplay, 0), DefaultDepth(pDisplay, 0), ZPixmap, 0, pData, width, height, 32, 0);
XEvent event;
while (XPending(pDisplay))
{
XNextEvent(pDisplay, &event);
if (event.type == Expose)
{
XPutImage(pDisplay, window, DefaultGC(pDisplay, 0), pImage, 0, 0, 0, 0, width, height);
}
}
}
}
// Cleanup
for (uint32_t i = 0; i < NUM_BUF; i++)
{
GevFreeImageBuffer(hCamera, pBuffer[i]);
free(pBuffer[i]);
}
XCloseDisplay(pDisplay);
GevStopImageTransfer(hCamera);
GevCloseCamera(&hCamera);
GevApiUninitialize();
return EXIT_SUCCESS;
}
```
注意:这只是一个示例代码,需要根据具体的相机型号和网络环境进行适当的修改。
相关推荐









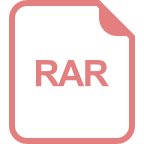