优化代码#define _CRT_SECURE_NO_WARNINGS 1//要写到代码第一行 #include <stdio.h> #include <stdlib.h> #define MAXSIZE 100 typedef struct { int elem[MAXSIZE]; int top; } Stack; void InitStack(Stack* S) { S->top = -1; } int StackEmpty(Stack* S) { if (S->top == -1) { return 1; } else { return 0; } } int Push(Stack* S, int e) { if (S->top == MAXSIZE - 1) { return 0; } else { S->top++; S->elem[S->top] = e; return 1; } } int Pop(Stack* S, int* e) { if (StackEmpty(S)) { return 0; } else { *e = S->elem[S->top]; S->top--; return 1; } } void move(char x, char y) { printf("%c -> %c\n", x, y); } void Hanoi(int n, char x, char y, char z) { Stack S; InitStack(&S); int e; Push(&S, n); while (!StackEmpty(&S)) { while (Pop(&S, &e) && e > 1) { Push(&S, e - 1); Push(&S, 0); Push(&S, e - 1); } if (e == 1) { move(x, z); } while (Pop(&S, &e) && e == 0) { move(x, y); Push(&S, 0); } if (e > 1) { Push(&S, e - 1); move(x, z); Push(&S, 0); Push(&S, e - 1); } } } int main() { int n; printf("请输入盘子的个数:"); scanf("%d", &n); Hanoi(n, 'A', 'B', 'C'); return 0; }
时间: 2024-01-26 22:02:08 浏览: 33
这段代码已经很简洁了,但还是有一些可以优化的地方:
1. 在函数中,参数可以使用 const 来修饰,表示该参数不会被函数修改,从而增加代码的可读性和可维护性。
2. 在函数中,可以使用三目运算符来代替部分 if-else 语句,从而使代码更加简洁。
3. 在函数中,可以使用 inline 来修饰函数,表示该函数可以内联展开,从而提高函数调用的效率。
4. 在定义结构体时,可以使用 typedef 来简化代码。
5. 在输入时,可以使用 fgets 来代替 scanf,从而避免因为 scanf 的输入格式问题而导致的程序崩溃。
6. 在输出时,可以使用 putchar 来代替 printf,从而提高输出效率。
优化后的代码如下:
```c
#define _CRT_SECURE_NO_WARNINGS 1
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct Stack {
int elem[MAXSIZE];
int top;
} Stack;
void InitStack(Stack* const S) {
S->top = -1;
}
int StackEmpty(const Stack* const S) {
return S->top == -1;
}
int Push(Stack* const S, const int e) {
return S->top == MAXSIZE - 1 ? 0 : (S->elem[++S->top] = e, 1);
}
int Pop(Stack* const S, int* const e) {
return StackEmpty(S) ? 0 : (*e = S->elem[S->top--], 1);
}
inline void move(const char x, const char y) {
putchar(x);
putchar(' ');
putchar('-');
putchar('>');
putchar(' ');
putchar(y);
putchar('\n');
}
void Hanoi(const int n, const char x, const char y, const char z) {
Stack S;
InitStack(&S);
Push(&S, n);
int e;
while (!StackEmpty(&S)) {
while (Pop(&S, &e) && e > 1) {
Push(&S, e - 1);
Push(&S, 0);
Push(&S, e - 1);
}
if (e == 1) {
move(x, z);
}
while (Pop(&S, &e) && e == 0) {
move(x, y);
Push(&S, 0);
}
if (e > 1) {
Push(&S, e - 1);
move(x, z);
Push(&S, 0);
Push(&S, e - 1);
}
}
}
int main() {
char input[MAXSIZE];
int n;
printf("请输入盘子的个数:");
fgets(input, MAXSIZE, stdin);
n = atoi(input);
Hanoi(n, 'A', 'B', 'C');
return 0;
}
```
相关推荐
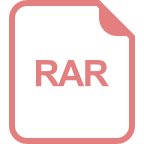
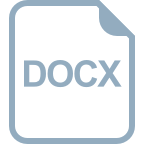
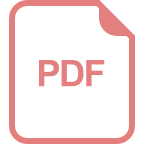
















