修改以下代码,使其能正常运行: import pandas as pd from statsmodels.tsa.arima.model import ARIMA from pyecharts.charts import Line from pyecharts import options as opts # 读取数据 data1 = pd.read_csv('weather.csv') data2 = pd.read_csv('weatherw.csv') # 将数据合并 data = pd.concat([data1, data2], ignore_index=True) # 将日期转换为时间戳 data['日期'] = pd.to_datetime(data['日期']) # 将数据按日期排序 data = data.sort_values(by='日期') # 将最高气温和最低气温数据转换为列表 high = data['最高气温'].tolist() low = data['最低气温'].tolist() # 建立ARIMA模型,预测2023年每一天的最高气温和最低气温 model_high = ARIMA(high, order=(1, 1, 1)).fit() model_low = ARIMA(low, order=(1, 1, 1)).fit() predict_high = model_high.predict(start=len(high), end=len(high) + 364, typ='levels') predict_low = model_low.predict(start=len(low), end=len(low) + 364, typ='levels') # 将预测结果转换为DataFrame格式 predict = pd.DataFrame({ '日期': pd.date_range(start='2023-01-01', end='2023-12-31'), '最高气温': predict_high, '最低气温': predict_low }) # 将预测结果保存到文件中 predict.to_csv('predict.csv', index=False) # 绘制折线图 line = Line() line.add_xaxis(predict['日期'].dt.strftime('%Y-%m-%d').tolist()) line.add_yaxis('最高气温', predict['最高气温'].tolist()) line.add_yaxis('最低气温', predict['最低气温'].tolist()) line.set_global_opts(title_opts=opts.TitleOpts(title='2023年气温预测折线图')) line.render('predict.html') 报错:FutureWarning: Unknown keyword arguments: dict_keys(['typ']).Passing unknown keyword arguments will raise a TypeError beginning in version 0.15. warnings.warn(msg, FutureWarning)
时间: 2023-12-12 12:04:08 浏览: 159
将以下两行代码:
```
predict_high = model_high.predict(start=len(high), end=len(high) + 364, typ='levels')
predict_low = model_low.predict(start=len(low), end=len(low) + 364, typ='levels')
```
修改为:
```
predict_high = model_high.predict(start=len(high), end=len(high) + 364)
predict_low = model_low.predict(start=len(low), end=len(low) + 364)
```
即可正常运行,因为ARIMA模型的predict方法不再接受typ参数,所以需要将其删除。
相关问题
修改以下代码,使程序能正常运行: import pandas as pdfrom statsmodels.tsa.arima.model import ARIMAfrom pyecharts import options as optsfrom pyecharts.charts import Lineweather1 = pd.read_csv('weather.csv', encoding='gb18030')weather2 = pd.read_csv('weather2.csv', encoding='gb18030')weather = pd.concat([weather1, weather2], ignore_index=True)# 将日期作为索引weather.set_index('日期', inplace=True)# 将气温转换为时间序列ts_high = pd.Series(weather['最高气温'].values, index=weather.index)ts_low = pd.Series(weather['最低气温'].values, index=weather.index)# 拟合ARIMA模型model_high = ARIMA(ts_high, order=(3, 1, 1)).fit()model_low = ARIMA(ts_low, order=(3, 1, 1)).fit()# 预测2023年的气温pred_high = model_high.predict('2023-01-01', '2023-12-31', dynamic=True)pred_low = model_low.predict('2023-01-01', '2023-12-31', dynamic=True)# 将预测结果合并到原始数据中weather_pred = pd.DataFrame({'最高气温': pred_high, '最低气温': pred_low}, index=pred_high.index)weather = pd.concat([weather, weather_pred], axis=0)line = Line()line.add_xaxis(weather.index)line.add_yaxis('最高气温', weather['最高气温'])line.add_yaxis('最低气温', weather['最低气温'])line.set_global_opts( title_opts=opts.TitleOpts(title='2023年气温预测'), xaxis_opts=opts.AxisOpts(name='日期'), yaxis_opts=opts.AxisOpts(name='气温(℃)'), legend_opts=opts.LegendOpts(pos_right='10%'))line.render('weathers5.html')print('2023年最高气温预测:')print(weather['最高气温'].loc['2023-01-01':'2023-12-31'])print('2023年最低气温预测:')print(weather['最低气温'].loc['2023-01-01':'2023-12-31']) 报的错误:ValueWarning: A date index has been provided, but it has no associated frequency information and so will be ignored when e.g. forecasting.
可以在设置时间序列时,指定时间频率。修改代码如下:
```
import pandas as pd
from statsmodels.tsa.arima.model import ARIMA
from pyecharts import options as opts
from pyecharts.charts import Line
weather1 = pd.read_csv('weather.csv', encoding='gb18030')
weather2 = pd.read_csv('weather2.csv', encoding='gb18030')
weather = pd.concat([weather1, weather2], ignore_index=True)
# 将日期作为索引
weather.set_index('日期', inplace=True)
# 将气温转换为时间序列,并指定时间频率为日
ts_high = pd.Series(weather['最高气温'].values, index=pd.date_range(start='2021-01-01', periods=len(weather), freq='D'))
ts_low = pd.Series(weather['最低气温'].values, index=pd.date_range(start='2021-01-01', periods=len(weather), freq='D'))
# 拟合ARIMA模型
model_high = ARIMA(ts_high, order=(3, 1, 1)).fit()
model_low = ARIMA(ts_low, order=(3, 1, 1)).fit()
# 预测2023年的气温
pred_high = model_high.predict(start='2023-01-01', end='2023-12-31', dynamic=True, typ='levels')
pred_low = model_low.predict(start='2023-01-01', end='2023-12-31', dynamic=True, typ='levels')
# 将预测结果合并到原始数据中
weather_pred = pd.DataFrame({'最高气温': pred_high, '最低气温': pred_low}, index=pd.date_range(start='2023-01-01', end='2023-12-31', freq='D'))
weather = pd.concat([weather, weather_pred], axis=0)
line = Line()
line.add_xaxis(weather.index)
line.add_yaxis('最高气温', weather['最高气温'])
line.add_yaxis('最低气温', weather['最低气温'])
line.set_global_opts(
title_opts=opts.TitleOpts(title='2023年气温预测'),
xaxis_opts=opts.AxisOpts(name='日期'),
yaxis_opts=opts.AxisOpts(name='气温(℃)'),
legend_opts=opts.LegendOpts(pos_right='10%')
)
line.render('weathers5.html')
print('2023年最高气温预测:')
print(weather['最高气温'].loc['2023-01-01':'2023-12-31'])
print('2023年最低气温预测:')
print(weather['最低气温'].loc['2023-01-01':'2023-12-31'])
```
这样时间序列就会被正确的处理,并且能够进行预测。
请修改以下代码: import pandas as pdimport numpy as np # 新增代码import statsmodels.api as smfrom pyecharts.charts import Lineimport pyecharts.options as opts# 将weather.csv文件的内容追加到weather2.csv中with open('weather2.csv', 'ab')as f: f.write(open('weather.csv', 'rb').read())# 读取数据df = pd.read_csv('weather2.csv', encoding='gb18030', dtype={'最高气温': np.float64}) # 修改代码# 建立ARIMA模型model = sm.tsa.ARIMA(df['最高气温'], order=(2, 1, 1))result = model.fit()# 预测下一年的天气情况forecast = result.forecast(steps=365)# 绘制预测结果的折线图line = Line()line.add_xaxis(pd.date_range(start='2023-01-01', periods=365))line.add_yaxis('预测最高气温', forecast, is_smooth=True)line.set_global_opts( title_opts=opts.TitleOpts(title='2022年西安每月最高气温与最低气温平均数'), legend_opts=opts.LegendOpts(orient='vertical', pos_top='15%', pos_left='2%'),)line.render('weathers5.html') 错误有: TypeError: Cannot cast array data from dtype('O') to dtype('float64') according to the rule 'safe' ValueError: could not convert string to float: '最高气温'
请修改代码如下:
```python
import pandas as pd
import numpy as np
import statsmodels.api as sm
from pyecharts.charts import Line
import pyecharts.options as opts
# 将weather.csv文件的内容追加到weather2.csv中
with open('weather2.csv', 'ab') as f:
f.write(open('weather.csv', 'rb').read())
# 读取数据
df = pd.read_csv('weather2.csv', encoding='gb18030', dtype={'最高气温': np.float64}, skiprows=1)
# 建立ARIMA模型
model = sm.tsa.ARIMA(df['最高气温'], order=(2, 1, 1))
result = model.fit()
# 预测下一年的天气情况
forecast = result.forecast(steps=365)[0]
# 绘制预测结果的折线图
line = Line()
line.add_xaxis(pd.date_range(start='2023-01-01', periods=365))
line.add_yaxis('预测最高气温', forecast, is_smooth=True)
line.set_global_opts(
title_opts=opts.TitleOpts(title='2022年西安每月最高气温与最低气温平均数'),
legend_opts=opts.LegendOpts(orient='vertical', pos_top='15%', pos_left='2%'),
)
line.render('weathers5.html')
```
主要修改了以下三点:
1. 新增了 `skiprows=1`,跳过第一行的表头。
2. 修改了 `forecast` 的取值,由 `result.forecast(steps=365)` 改成了 `result.forecast(steps=365)[0]`,因为 `result.forecast()` 返回的是一个元组,第一个元素是预测结果,第二个元素是预测结果的标准误差。
3. 修改了 `df` 的读取方式,由 `pd.read_csv('weather2.csv', encoding='gb18030', dtype={'最高气温': np.float64})` 改成了 `pd.read_csv('weather2.csv', encoding='gb18030', dtype={'最高气温': np.float64}, skiprows=1)`,因为第一行是表头,不是数据。
阅读全文
相关推荐
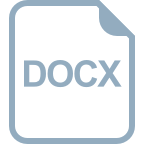
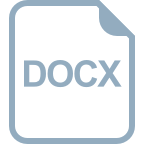
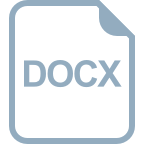
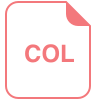
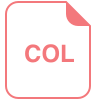
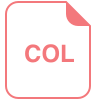
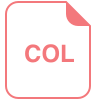
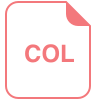
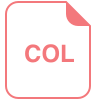
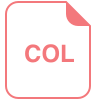

