用akshare,gym写出股票强化学习代码
时间: 2023-06-30 21:16:27 浏览: 53
好的,我可以为您提供一个简单的股票强化学习代码,使用akshare和gym库。以下是代码:
```python
import gym
import akshare as ak
import numpy as np
# 获取股票数据
stock_code = 'sh600000'
stock_df = ak.stock_zh_a_hist(stock_code)
# 定义股票强化学习环境
class StockEnv(gym.Env):
metadata = {'render.modes': ['human']}
def __init__(self, data, initial_investment=20000):
super(StockEnv, self).__init__()
self.data = data
self.initial_investment = initial_investment
self.action_space = gym.spaces.Discrete(3) # 买入、卖出、不操作
self.observation_space = gym.spaces.Box(low=0, high=1, shape=(6,))
self.reset()
def reset(self):
self.current_step = 0
self.balance = self.initial_investment
self.shares = 0
self.net_worth = self.balance + self.shares * self.data[self.current_step][3]
return self._next_observation()
def _next_observation(self):
obs = np.array([
self.data[self.current_step][1] / max(self.data[:, 1]), # 当前股价
self.data[self.current_step][2] / max(self.data[:, 2]), # 最高股价
self.data[self.current_step][3] / max(self.data[:, 3]), # 最低股价
self.data[self.current_step][4] / max(self.data[:, 4]), # 当前成交量
self.balance / self.net_worth, # 当前账户余额占净值的比例
self.shares / self.net_worth # 当前持有股票价值占净值的比例
])
return obs
def step(self, action):
assert self.action_space.contains(action)
prev_net_worth = self.net_worth
current_price = self.data[self.current_step][3]
if action == 0: # 买入股票
shares_to_buy = int(self.balance / current_price)
self.shares += shares_to_buy
self.balance -= shares_to_buy * current_price
elif action == 1: # 卖出股票
shares_to_sell = self.shares
self.shares = 0
self.balance += shares_to_sell * current_price
self.current_step += 1
self.net_worth = self.balance + self.shares * current_price
reward = self.net_worth - prev_net_worth
done = self.current_step == len(self.data) - 1
return self._next_observation(), reward, done, {}
def render(self, mode='human'):
print(f'Step: {self.current_step}')
print(f'Balance: {self.balance}')
print(f'Shares: {self.shares}')
print(f'Net Worth: {self.net_worth}')
# 定义股票交易策略
def policy(obs):
if obs[0] > obs[1]: # 股价上涨,买入股票
return 0
elif obs[0] < obs[1]: # 股价下跌,卖出股票
return 1
else: # 股价不变,不操作
return 2
# 训练股票强化学习模型
env = StockEnv(stock_df.values)
for episode in range(10):
obs = env.reset()
done = False
while not done:
action = policy(obs)
obs, reward, done, info = env.step(action)
env.render()
```
该代码通过akshare库获取股票数据,定义了一个股票强化学习环境,并在该环境上实现了一个简单的股票交易策略。在训练过程中,模型将根据当前观察到的股票数据来决定下一步应该采取的行动,直到达到指定的训练次数。
相关推荐
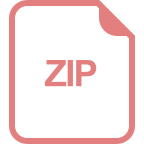
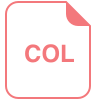
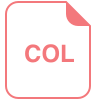
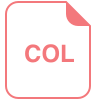










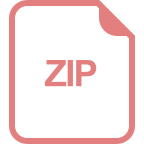