BertClassfication( (model): BertModel( (embeddings): BertEmbeddings( (word_embeddings): Embedding(21128, 768, padding_idx=0) (position_embeddings): Embedding(512, 768) (token_type_embeddings): Embedding(2, 768) (LayerNorm): LayerNorm((768,), eps=1e-12, elementwise_affine=True) (dropout): Dropout(p=0.1, inplace=False) ) (encoder): BertEncoder( (layer): ModuleList( (0-11): 12 x BertLayer( (attention): BertAttention( (self): BertSelfAttention( (query): Linear(in_features=768, out_features=768, bias=True) (key): Linear(in_features=768, out_features=768, bias=True) (value): Linear(in_features=768, out_features=768, bias=True) (dropout): Dropout(p=0.1, inplace=False) ) (output): BertSelfOutput( (dense): Linear(in_features=768, out_features=768, bias=True) (LayerNorm): LayerNorm((768,), eps=1e-12, elementwise_affine=True) (dropout): Dropout(p=0.1, inplace=False) ) ) (intermediate): BertIntermediate( (dense): Linear(in_features=768, out_features=3072, bias=True) (intermediate_act_fn): GELUActivation() ) (output): BertOutput( (dense): Linear(in_features=3072, out_features=768, bias=True) (LayerNorm): LayerNorm((768,), eps=1e-12, elementwise_affine=True) (dropout): Dropout(p=0.1, inplace=False) ) ) ) ) (pooler): BertPooler( (dense): Linear(in_features=768, out_features=768, bias=True) (activation): Tanh() ) ) (fc): Linear(in_features=768, out_features=15, bias=True) )解读此模型
时间: 2023-06-04 20:05:38 浏览: 154
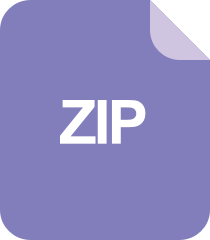
bio_embeddings:从蛋白质序列中获取蛋白质嵌入

这是一个Bert模型与线性分类器结合而成的模型,用于文本分类任务。模型中包含了Bert的基本结构,包括嵌入层、编码器、池化器等,其中编码器由12个BertLayer组成,每个BertLayer包含了多头自注意力机制、前馈层等子模块。在Bert编码器的输出上,采用了池化层BertPooler,将整个句子的信息压缩成一个向量表示。最后,通过一个线性分类器将特征映射到15个类别。这个模型可以用于文本分类任务,例如情感分析、垃圾邮件检测等。
阅读全文
相关推荐
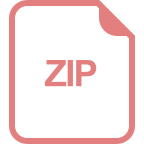
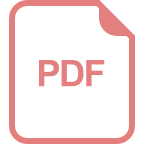















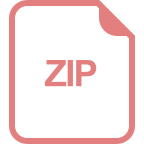