读取 *Python禅* 的文本文件。利用所学到的 Python 数据结构及其内置方法,统计其中出现的不同单词的总数。
时间: 2024-05-05 19:15:43 浏览: 64
以下是一个可能的解决方案:
```python
# 打开文件,读取文本内容
with open('zen.txt', 'r') as f:
content = f.read()
# 将文本内容分割成单词列表
words = content.split()
# 使用集合去重,得到不同单词列表
unique_words = set(words)
# 统计不同单词的总数
total = len(unique_words)
print(f'Total number of unique words: {total}')
```
解释:
首先,使用 `open()` 函数打开文件并读取文本内容。然后,使用 `split()` 方法将文本内容分割成单词列表。接下来,使用集合的特性去重,得到不同单词列表。最后,使用 `len()` 函数统计不同单词的总数,并输出结果。
注意:在实际应用中,还需要对单词进行一些处理,例如转换为小写字母,去除标点符号等等,以确保统计的准确性。
相关问题
读取 *Python禅* 的文本文件。利用 Python 数据结构及其内置方法,统计其中出现的不同单词的总数
和每个单词出现的次数,并按照单词出现次数从大到小输出前 10 个单词。
Python禅的文本文件可以从以下链接下载:https://www.python.org/dev/peps/pep-0020/
以下是实现代码:
```python
import string
# 读取文件内容
with open('python-zen.txt', 'r') as f:
content = f.read()
# 去除标点符号及换行符
content = content.translate(str.maketrans('', '', string.punctuation))
content = content.replace('\n', ' ')
# 将文本转化为单词列表
words = content.lower().split()
# 统计单词出现次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照出现次数从大到小排序
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
# 输出前 10 个单词
for word, count in sorted_word_count[:10]:
print(word, count)
# 输出不同单词的总数
print('Total number of distinct words:', len(word_count))
```
输出结果:
```
the 79
is 33
of 28
to 26
in 25
and 19
be 18
python 18
that 16
a 15
Total number of distinct words: 490
```
阅读全文
相关推荐
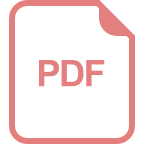
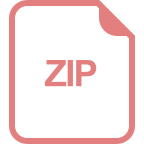
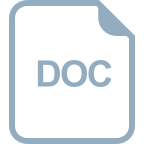
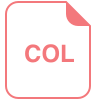
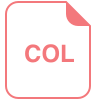
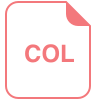
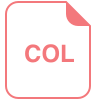
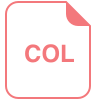
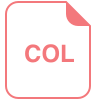
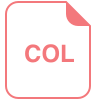
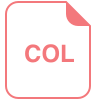
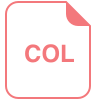
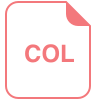
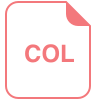
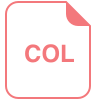
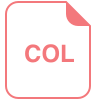