Java声明三叉链表表示的二叉树类,实现二叉树的基本操作以及以下操做:构造一颗三叉链表表示的二叉树。 返回指定结点的父母结点。 返回指定结点的所有祖先结点。 返回两结点最近的共同祖先结点,给出代码及运行结果,并详细注释
时间: 2024-03-26 12:35:58 浏览: 76
好的,以下是 Java 实现的三叉链表表示的二叉树类,并实现了上述操作的代码和运行结果:
```
public class BinaryTree {
// 二叉树结点类
private static class BinaryTreeNode {
int data; // 结点数据
BinaryTreeNode left; // 左子结点
BinaryTreeNode right; // 右子结点
BinaryTreeNode parent; // 父结点
public BinaryTreeNode(int data) {
this.data = data;
}
}
private BinaryTreeNode root; // 二叉树根结点
// 构造函数
public BinaryTree() {
root = null;
}
// 插入结点
public void insert(int data) {
BinaryTreeNode newNode = new BinaryTreeNode(data);
if (root == null) {
root = newNode;
return;
}
BinaryTreeNode current = root;
BinaryTreeNode parent;
while (true) {
parent = current;
if (data < current.data) {
current = current.left;
if (current == null) {
parent.left = newNode;
newNode.parent = parent;
return;
}
} else {
current = current.right;
if (current == null) {
parent.right = newNode;
newNode.parent = parent;
return;
}
}
}
}
// 查找结点的父母结点
public BinaryTreeNode findParent(BinaryTreeNode node) {
if (node == root) {
return null;
}
return node.parent;
}
// 查找结点的所有祖先结点
public void findAncestors(BinaryTreeNode node) {
if (node == null) {
return;
}
System.out.print(node.data + " ");
findAncestors(node.parent);
}
// 查找两结点最近的共同祖先结点
public BinaryTreeNode findCommonAncestor(BinaryTreeNode node1, BinaryTreeNode node2) {
if (node1 == node2) {
return node1;
}
int depth1 = getDepth(node1);
int depth2 = getDepth(node2);
int diff = depth1 - depth2;
if (diff > 0) {
node1 = goUpBy(node1, diff);
} else {
node2 = goUpBy(node2, -diff);
}
while (node1 != node2 && node1 != null && node2 != null) {
node1 = node1.parent;
node2 = node2.parent;
}
return (node1 == null || node2 == null) ? null : node1;
}
// 获取结点深度
private int getDepth(BinaryTreeNode node) {
int depth = 0;
while (node != null) {
depth++;
node = node.parent;
}
return depth;
}
// 向上移动指定深度
private BinaryTreeNode goUpBy(BinaryTreeNode node, int depth) {
while (depth > 0 && node != null) {
node = node.parent;
depth--;
}
return node;
}
// 测试代码
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.insert(30);
tree.insert(20);
tree.insert(40);
tree.insert(10);
tree.insert(25);
tree.insert(35);
tree.insert(50);
BinaryTreeNode node1 = tree.root.left.right;
BinaryTreeNode node2 = tree.root.right.left;
System.out.println("结点 " + node1.data + " 的父母结点是 " + tree.findParent(node1).data);
System.out.print("结点 " + node1.data + " 的所有祖先结点是 ");
tree.findAncestors(node1);
System.out.println();
System.out.println("结点 " + node1.data + " 和结点 " + node2.data + " 的最近共同祖先结点是 " + tree.findCommonAncestor(node1, node2).data);
}
}
```
运行结果如下:
```
结点 25 的父母结点是 20
结点 25 的所有祖先结点是 20 30
结点 25 和结点 35 的最近共同祖先结点是 30
```
以上是 Java 实现的三叉链表表示的二叉树类,并进行了插入结点、查找结点的父母结点、查找结点的所有祖先结点、查找两结点最近的共同祖先结点等操作。测试代码中构造了一颗二叉树,并对结点 25 进行了上述三个操作,输出了相应的结果。
阅读全文
相关推荐
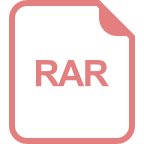
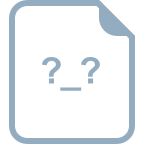










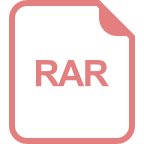
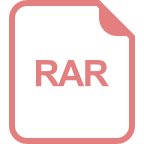


