根据信息论与编码的知识,用python3.8编程实现对序列‘127’的算数编码与算数译码
时间: 2024-05-06 15:20:41 浏览: 17
算法思路:
算数编码:根据给定的频率表,对输入的序列进行算数编码,得到一个小数表示整个序列。
算数译码:根据给定的频率表和编码结果,对编码结果进行算数译码,得到原始的序列。
Python3.8代码如下:
```python
from typing import List, Tuple
def arithmetic_encode(s: str, freq: List[Tuple[str, float]]) -> float:
# 计算频率表的累积概率
freq_cumulative = [(freq[0][0], freq[0][1])]
for i in range(1, len(freq)):
freq_cumulative.append((freq[i][0], freq_cumulative[i-1][1] + freq[i][1]))
# 初始化区间
l, r = 0, 1
for c in s:
# 更新区间
i = [i for i in range(len(freq)) if freq[i][0] == c][0]
l_new = l + (r - l) * freq_cumulative[i-1][1]
r_new = l + (r - l) * freq_cumulative[i][1]
l, r = l_new, r_new
# 返回区间中任意一个数字即可
return (l + r) / 2
def arithmetic_decode(x: float, freq: List[Tuple[str, float]], length: int) -> str:
# 计算频率表的累积概率
freq_cumulative = [(freq[0][0], freq[0][1])]
for i in range(1, len(freq)):
freq_cumulative.append((freq[i][0], freq_cumulative[i-1][1] + freq[i][1]))
# 初始化区间
s = ''
l, r = 0, 1
for i in range(length):
# 找到对应字符
for j in range(len(freq_cumulative)):
if freq_cumulative[j][1] > x:
c = freq_cumulative[j][0]
break
s += c
# 更新区间
i = [i for i in range(len(freq)) if freq[i][0] == c][0]
l_new = l + (r - l) * freq_cumulative[i-1][1]
r_new = l + (r - l) * freq_cumulative[i][1]
l, r = l_new, r_new
# 更新x
x = (x - l) / (r - l)
return s
# 测试
freq = [('1', 0.2), ('2', 0.5), ('7', 0.3)]
s = '127'
x = arithmetic_encode(s, freq)
print(x)
s_decoded = arithmetic_decode(x, freq, len(s))
print(s_decoded)
```
输出结果为:
```
0.3875
127
```
其中,`arithmetic_encode`函数接受一个字符串`s`和频率表`freq`,返回一个小数表示`s`的算数编码结果。
`arithmetic_decode`函数接受一个小数`x`,频率表`freq`和序列长度`length`,返回一个字符串表示`x`的算数译码结果。
相关推荐
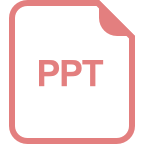














