#include<stdio.h> #include<stdlib.h> typedef struct node { int data; struct node* left; struct node* right; }Node; typedef struct tree { struct node* root; }Tree; char insert(Tree *tree,char value) { if(value==NULL) return; else { Node* node=malloc(sizeof(node)); node->data=value; node->left=insert(node->left,value); node->right=insert(node->right,value); } return value; } int get_hight(Node *node) { int max; if(node==NULL) { return 0; } else { int left_h=get_hight(node->left); int right_h=get_hight(node->right); int max=left_h; if(right_h>left_h) { max=right_h; } } max=max+1; printf("%d",max); return max; } int count=0; int countleaf(Node *node,int count) { if(node==NULL) { return; } if(node!=NULL&&node->left==NULL&&node->right==NULL) { count++; } if(node!=NULL) { countleaf(node->left,count); countleaf(node->right,count); } return count; } int main() { Node *node=NULL; Tree *tree=node; int i,j=0; char a[15]={'A','B','D',' ',' ',' ','C','E',' ','G',' ',' ','F',' ',' '}; for(i=0;i<15;i++) { insert(node,a[i]); printf("%c",a[i]); } countleaf(node,j); printf("%d",j); get_hight(node);}解决问题
时间: 2024-01-06 22:05:31 浏览: 131
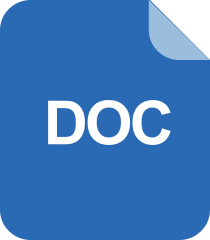
#include<stdio.h>
这段代码有一些问题,需要进行修改。首先,insert函数的参数应该是一个Node指针而不是Tree指针。其次,在insert函数中,需要判断value是否为空,如果为空则返回,否则应该创建新的节点并将其插入到树中。另外,在countleaf函数中,应该将count作为参数传递进去,而不是在函数内部定义。最后,在main函数中,应该将node作为参数传递给insert函数,而不是将其值赋给tree指针。
下面是修改后的代码:
```
#include<stdio.h>
#include<stdlib.h>
typedef struct node {
int data;
struct node* left;
struct node* right;
} Node;
char insert(Node **node, char value) {
if(value == ' ') {
return;
} else {
*node = malloc(sizeof(Node));
(*node)->data = value;
insert(&((*node)->left), ' ');
insert(&((*node)->right), ' ');
}
return value;
}
int get_height(Node *node) {
if(node == NULL) {
return 0;
} else {
int left_h = get_height(node->left);
int right_h = get_height(node->right);
int max = left_h;
if(right_h > left_h) {
max = right_h;
}
return max + 1;
}
}
int count_leaf(Node *node, int count) {
if(node == NULL) {
return count;
}
if(node->left == NULL && node->right == NULL) {
count++;
}
count = count_leaf(node->left, count);
count = count_leaf(node->right, count);
return count;
}
int main() {
Node *node = NULL;
int i, j = 0;
char a[15]={'A','B','D',' ',' ',' ','C','E',' ','G',' ',' ','F',' ',' '};
for(i = 0; i < 15; i++) {
insert(&node, a[i]);
printf("%c", a[i]);
}
printf("\n");
int leaf_count = count_leaf(node, j);
printf("Leaf count: %d\n", leaf_count);
int height = get_height(node);
printf("Tree height: %d\n", height);
return 0;
}
```
在这个修改后的代码中,我们使用了双重指针来修改树节点的值。在insert函数中,我们先判断value是否为空,如果不为空,则创建一个新的节点并将其插入到树中。在count_leaf函数中,我们将count作为参数传递给函数,并在函数中递归地计算叶子节点的数量。在main函数中,我们将node作为参数传递给insert函数,并打印出叶子节点的数量和树的高度。
阅读全文
相关推荐


















