#include <stdio.h> #include <stdlib.h> typedef int E; struct ListNode{ E element; // 保存当前元素 struct ListNode *next; // 指向下一个节点的指针 }; typedef struct ListNode* Node; 以此段代码为基础编写一个带表头和表尾两个指针的单循环链表
时间: 2024-04-10 22:27:59 浏览: 20
基于这段代码,我们可以编写一个带表头和表尾两个指针的单循环链表。下面是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef int E;
struct ListNode{
E element; // 保存当前元素
struct ListNode *next; // 指向下一个节点的指针
};
typedef struct ListNode* Node;
// 创建一个带表头和表尾指针的单循环链表
typedef struct {
Node head; // 表头指针
Node tail; // 表尾指针
} CircularLinkedList;
// 初始化链表
void initList(CircularLinkedList* list) {
list->head = NULL;
list->tail = NULL;
}
// 在链表末尾插入元素
void insert(CircularLinkedList* list, E element) {
Node newNode = (Node)malloc(sizeof(struct ListNode));
newNode->element = element;
if (list->head == NULL) {
newNode->next = newNode; // 新节点指向自身形成循环链表
list->head = newNode;
list->tail = newNode;
} else {
newNode->next = list->head; // 新节点指向头结点
list->tail->next = newNode; // 表尾指针指向新节点
list->tail = newNode; // 更新表尾指针
}
}
// 打印链表元素
void printList(CircularLinkedList* list) {
if (list->head == NULL) {
printf("List is empty.\n");
return;
}
Node current = list->head;
do {
printf("%d ", current->element);
current = current->next;
} while (current != list->head);
printf("\n");
}
// 释放链表内存
void freeList(CircularLinkedList* list) {
if (list->head == NULL) {
return;
}
Node current = list->head;
while (current != list->tail) {
Node temp = current;
current = current->next;
free(temp);
}
free(list->tail);
list->head = NULL;
list->tail = NULL;
}
int main() {
CircularLinkedList list;
initList(&list);
insert(&list, 1);
insert(&list, 2);
insert(&list, 3);
printList(&list);
freeList(&list);
return 0;
}
```
这个示例代码实现了一个带表头和表尾指针的单循环链表,并且包括了初始化链表、在末尾插入元素、打印链表元素和释放链表内存的功能。在主函数中,我们创建一个链表,插入一些元素,并打印链表的内容,最后释放链表内存。你可以根据需要修改和扩展这个代码以满足具体的需求。
相关推荐
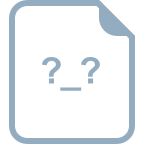
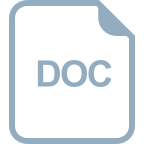
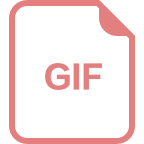














