检查代码问题#include <stdio.h> #include <stdlib.h> typedef struct ListNode { int val; struct ListNode* next; } ListNode; ListNode* createList(int n) { ListNode* head = NULL; ListNode* tail = NULL; ListNode* p = NULL; for (int i = 0; i < n; i++) { p = (ListNode*)malloc(sizeof(ListNode)); scanf("%d", &(p->val)); p->next = NULL; if (head == NULL) { head = p; tail = p; } else { tail->next = p; tail = p; } } return head; } ListNode* removeElements(ListNode* head, int val) { ListNode* dummy = (ListNode*)malloc(sizeof(ListNode)); dummy->val = 0; dummy->next = head; ListNode* p = dummy; while (p->next) { if (p->next->val == val) { ListNode* tmp = p->next; p->next = tmp->next; free(tmp); } else { p = p->next; } } head = dummy->next; free(dummy); return head; } void printList(ListNode* head) { while (head) { printf("%d ", head->val); head = head->next; } } int main() { int n, val; scanf("%d", &n); ListNode* head = createList(n); scanf("%d", &val); head = removeElements(head, val); printList(head); return 0; }
时间: 2023-06-19 11:03:41 浏览: 104
这段代码看起来没什么问题。但是有两个小的建议:
1. 在 `createList` 函数中,当分配内存时,应该检查指针是否为 NULL。如果分配失败,应该及时停止程序并返回错误。
2. 在 `removeElements` 函数中,dummy 节点的 `val` 域赋值为 0 是没有必要的,因为在删除链表元素时,不涉及到 `val` 域的值。
相关问题
帮我改一下代码的逻辑,这是以下代码:#include<stdio.h> #include<stdlib.h> struct ListNode { //链表结构体的重命名 int val; struct ListNode* next; }; typedef struct ListNode ListNode; ListNode * createList(); //创建链表函数 void releaseList(ListNode * head); //释放链表函数 int findMax(ListNode * head); //返回链表中的最大值函数 int findMin(ListNode * head); //返回链表中的最小值函数 int sum = 0; //记录和的变量 int main() { ListNode * head = createList(); printf("The maximum,minmum and the total are:%d %d %d\n", findMax(head), findMin(head), sum); releaseList(head); head = NULL; return 0; } ListNode * createList() { int num; ListNode *current = NULL; ListNode *last = NULL; ListNode head = NULL; scanf("%d", &num); while (num != -1) { current = (ListNode)malloc(sizeof(ListNode)); if (current != NULL) { current->val = num; sum += num; if (head == NULL) { head = current; last = current; } else { last->next = current; last = current; } } scanf("%d", &num); } if (last != NULL) { last->next = NULL; } return head; } void releaseList(ListNode * head) { ListNode * temp; while (head != NULL) { temp = head; head = head->next; free(temp); } } int findMax(ListNode * head) { ListNode * curr = head; int max_val = curr->val; while (curr != NULL) { if (curr->val > max_val) { max_val = curr->val; } curr = curr->next; } return max_val; } int findMin(ListNode * head) { ListNode * curr = head; int min_val = curr->val; while (curr != NULL) { if (curr->val < min_val) { min_val = curr->val; } curr = curr->next; } return min_val; }
以下是修改后的代码逻辑:
```
#include<stdio.h>
#include<stdlib.h>
struct ListNode {
int val;
struct ListNode* next;
};
typedef struct ListNode ListNode;
ListNode* createList();
void releaseList(ListNode* head);
int findMax(ListNode* head);
int findMin(ListNode* head);
int main() {
ListNode* head = createList();
int max_num = findMax(head);
int min_num = findMin(head);
int total_sum = 0;
ListNode* curr = head;
while (curr != NULL) {
total_sum += curr->val;
curr = curr->next;
}
printf("The maximum, minimum and total are: %d %d %d\n", max_num, min_num, total_sum);
releaseList(head);
head = NULL;
return 0;
}
ListNode* createList() {
int num;
ListNode* head = NULL;
ListNode* last = NULL;
scanf("%d", &num);
while (num != -1) {
ListNode* current = (ListNode*)malloc(sizeof(ListNode));
if (current != NULL) {
current->val = num;
current->next = NULL;
if (head == NULL) {
head = current;
last = current;
} else {
last->next = current;
last = current;
}
}
scanf("%d", &num);
}
return head;
}
void releaseList(ListNode* head) {
while (head != NULL) {
ListNode* temp = head;
head = head->next;
free(temp);
}
}
int findMax(ListNode* head) {
int max_num = head->val;
ListNode* curr = head->next;
while (curr != NULL) {
if (curr->val > max_num) {
max_num = curr->val;
}
curr = curr->next;
}
return max_num;
}
int findMin(ListNode* head) {
int min_num = head->val;
ListNode* curr = head->next;
while (curr != NULL) {
if (curr->val < min_num) {
min_num = curr->val;
}
curr = curr->next;
}
return min_num;
}
```
主要修改如下:
1. 在 `main` 函数中,先分别调用 `findMax` 和 `findMin` 函数找到最大值和最小值,然后再通过遍历链表计算总和,最后将这三个值一起输出。
2. 在 `createList` 函数中,将链表的头结点 `head` 初始化为 `NULL`,每次添加新节点时,都将该节点的 `next` 指针设置为 `NULL`,以确保链表的最后一个节点的 `next` 指针为 `NULL`。
3. 在 `releaseList` 函数中,不需要使用 `temp` 指针保存当前节点的地址,直接释放当前节点即可,因为当前节点的地址已经保存在 `head` 指针中了。
给你一个链表的头节点 head 和一个整数 val ,请你删除链表中所有满足 Node.val == val 的节点,并返回 新的头节点 head = [1,2,6,3,4,5,6], val = 6
要删除链表中所有值为 `val` 的节点,我们可以使用迭代或递归的方式遍历链表。这里我将提供一个使用迭代的方法:
首先,我们需要创建一个新的头节点 `new_head` 和两个指针 `prev` 和 `current`。`prev` 指针始终指向前一个节点,而 `current` 则用来遍历链表。
当遇到值等于 `val` 的节点时,我们将不将其添加到新的链表中,而是直接跳过它。如果当前节点不是要删除的,我们就将它的下一个节点赋给 `prev`,然后继续向前移动 `current`。当遍历完后,`prev` 就指向了新链表的最后一个节点(如果没有被删除的节点),所以我们把 `prev` 设置为新链表的头节点。
下面是相应的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
ListNode* removeElements(ListNode* head, int val) {
if (head == NULL || head->val != val) {
return head;
}
ListNode* new_head = NULL;
ListNode* prev = NULL;
ListNode* current = head;
while (current != NULL && current->val == val) {
current = current->next; // 直接跳过值为val的节点
}
if (current != NULL) { // 如果链表中有其他节点
prev = current;
current = current->next;
} else {
return NULL; // 如果链表只包含要删除的节点,则返回NULL
}
while (current != NULL) {
prev->next = current->next;
prev = prev->next;
if (prev->next == NULL) { // 遍历结束后,prev即为新链表的末尾
break;
}
if (prev->next->val == val) { // 内部循环检查并跳过剩余的val节点
prev->next = prev->next->next;
} else {
current = prev->next;
}
}
return new_head ? new_head : current; // 返回非空的新链表头节点
}
// 示例:
void printList(ListNode* node) {
while (node != NULL) {
printf("%d -> ", node->val);
node = node->next;
}
printf("NULL\n");
}
int main() {
ListNode* head = malloc(sizeof(ListNode));
head->val = 1;
head->next = malloc(sizeof(ListNode));
head->next->val = 2;
head->next->next = malloc(sizeof(ListNode));
head->next->next->val = 6;
head->next->next->next = malloc(sizeof(ListNode));
head->next->next->next->val = 3;
head->next->next->next->next = malloc(sizeof(ListNode));
head->next->next->next->next->val = 4;
head->next->next->next->next->next = malloc(sizeof(ListNode));
head->next->next->next->next->next->val = 5;
head->next->next->next->next->next->next = malloc(sizeof(ListNode));
head->next->next->next->next->next->next->val = 6;
head->next->next->next->next->next->next->next = NULL;
printf("Original list: ");
printList(head);
int valToRemove = 6;
ListNode* newHead = removeElements(head, valToRemove);
printf("New list after removing %d: ", valToRemove);
printList(newHead);
return 0;
}
```
运行这段代码后,你会看到原链表 `[1,2,6,3,4,5,6]` 删除所有 `6` 后的结果。如果你有其他关于这个操作的问题,或者需要帮助理解代码,请告诉我。
阅读全文
相关推荐
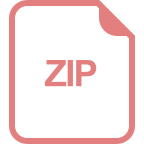
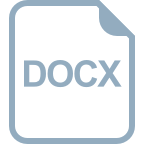
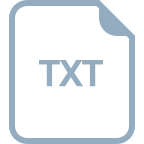













