帮我改一下代码的逻辑,这是以下代码:#include<stdio.h> #include<stdlib.h> struct ListNode { //链表结构体的重命名 int val; struct ListNode* next; }; typedef struct ListNode ListNode; ListNode * createList(); //创建链表函数 void releaseList(ListNode * head); //释放链表函数 int findMax(ListNode * head); //返回链表中的最大值函数 int findMin(ListNode * head); //返回链表中的最小值函数 int sum = 0; //记录和的变量 int main() { ListNode * head = createList(); printf("The maximum,minmum and the total are:%d %d %d\n", findMax(head), findMin(head), sum); releaseList(head); head = NULL; return 0; } ListNode * createList() { int num; ListNode *current = NULL; ListNode *last = NULL; ListNode head = NULL; scanf("%d", &num); while (num != -1) { current = (ListNode)malloc(sizeof(ListNode)); if (current != NULL) { current->val = num; sum += num; if (head == NULL) { head = current; last = current; } else { last->next = current; last = current; } } scanf("%d", &num); } if (last != NULL) { last->next = NULL; } return head; } void releaseList(ListNode * head) { ListNode * temp; while (head != NULL) { temp = head; head = head->next; free(temp); } } int findMax(ListNode * head) { ListNode * curr = head; int max_val = curr->val; while (curr != NULL) { if (curr->val > max_val) { max_val = curr->val; } curr = curr->next; } return max_val; } int findMin(ListNode * head) { ListNode * curr = head; int min_val = curr->val; while (curr != NULL) { if (curr->val < min_val) { min_val = curr->val; } curr = curr->next; } return min_val; }
时间: 2024-01-07 10:03:36 浏览: 81
以下是修改后的代码逻辑:
```
#include<stdio.h>
#include<stdlib.h>
struct ListNode {
int val;
struct ListNode* next;
};
typedef struct ListNode ListNode;
ListNode* createList();
void releaseList(ListNode* head);
int findMax(ListNode* head);
int findMin(ListNode* head);
int main() {
ListNode* head = createList();
int max_num = findMax(head);
int min_num = findMin(head);
int total_sum = 0;
ListNode* curr = head;
while (curr != NULL) {
total_sum += curr->val;
curr = curr->next;
}
printf("The maximum, minimum and total are: %d %d %d\n", max_num, min_num, total_sum);
releaseList(head);
head = NULL;
return 0;
}
ListNode* createList() {
int num;
ListNode* head = NULL;
ListNode* last = NULL;
scanf("%d", &num);
while (num != -1) {
ListNode* current = (ListNode*)malloc(sizeof(ListNode));
if (current != NULL) {
current->val = num;
current->next = NULL;
if (head == NULL) {
head = current;
last = current;
} else {
last->next = current;
last = current;
}
}
scanf("%d", &num);
}
return head;
}
void releaseList(ListNode* head) {
while (head != NULL) {
ListNode* temp = head;
head = head->next;
free(temp);
}
}
int findMax(ListNode* head) {
int max_num = head->val;
ListNode* curr = head->next;
while (curr != NULL) {
if (curr->val > max_num) {
max_num = curr->val;
}
curr = curr->next;
}
return max_num;
}
int findMin(ListNode* head) {
int min_num = head->val;
ListNode* curr = head->next;
while (curr != NULL) {
if (curr->val < min_num) {
min_num = curr->val;
}
curr = curr->next;
}
return min_num;
}
```
主要修改如下:
1. 在 `main` 函数中,先分别调用 `findMax` 和 `findMin` 函数找到最大值和最小值,然后再通过遍历链表计算总和,最后将这三个值一起输出。
2. 在 `createList` 函数中,将链表的头结点 `head` 初始化为 `NULL`,每次添加新节点时,都将该节点的 `next` 指针设置为 `NULL`,以确保链表的最后一个节点的 `next` 指针为 `NULL`。
3. 在 `releaseList` 函数中,不需要使用 `temp` 指针保存当前节点的地址,直接释放当前节点即可,因为当前节点的地址已经保存在 `head` 指针中了。
阅读全文
相关推荐
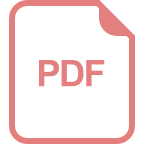
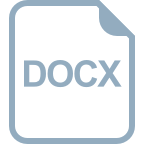
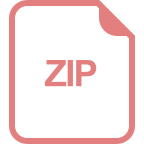














