补全如下代码:#include <stdio.h> #include <stdlib.h> struct ListNode { int data; struct ListNode *next; }; struct ListNode *readlist(); struct ListNode *deletem( struct ListNode *L, int m ); void printlist( struct ListNode *L ) { struct ListNode *p = L; while (p) { printf("%d ", p->data); p = p->next; } printf("\n"); } int main() { int m; struct ListNode *L = readlist(); scanf("%d", &m); L = deletem(L, m); printlist(L); return 0; }
时间: 2024-01-15 08:05:26 浏览: 30
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int data;
struct ListNode *next;
};
struct ListNode *readlist();
struct ListNode *deletem(struct ListNode *L, int m);
void printlist(struct ListNode *L);
int main() {
int m;
struct ListNode *L = readlist();
scanf("%d", &m);
L = deletem(L, m);
printlist(L);
return 0;
}
struct ListNode *readlist() {
struct ListNode *head = NULL, *tail = NULL;
int n, val;
scanf("%d", &n);
while (n--) {
scanf("%d", &val);
struct ListNode *node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->data = val;
node->next = NULL;
if (!head) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
struct ListNode *deletem(struct ListNode *L, int m) {
struct ListNode *head = NULL, *tail = NULL;
while (L) {
if (L->data != m) {
struct ListNode *node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->data = L->data;
node->next = NULL;
if (!head) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
L = L->next;
}
return head;
}
void printlist(struct ListNode *L) {
struct ListNode *p = L;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
该代码实现了链表的基本操作,包括创建链表、删除指定元素、打印链表等。其中,`readlist()`函数用于创建链表,`deletem()`函数用于删除指定元素,`printlist()`函数用于打印链表。主函数中,通过调用`readlist()`函数创建链表,并读入需要删除的元素,然后调用`deletem()`函数进行删除操作,最后调用`printlist()`函数打印删除后的链表。
相关推荐
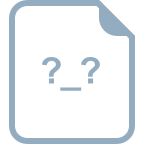
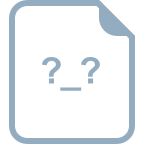














