#include <stdio.h> #include <stdlib.h> struct ListNode { int data; struct ListNode *next; }; struct ListNode *readlist(); struct ListNode *deletem( struct ListNode *L, int m ); void printlist( struct ListNode *L ) { struct ListNode *p = L; while (p) { printf("%d ", p->data); p = p->next; } printf("\n"); } int main() { int m; struct ListNode *L = readlist(); scanf("%d", &m); L = deletem(L, m); printlist(L); return 0; } /* 你的代码将被嵌在这里 */
时间: 2024-04-28 13:26:04 浏览: 36
以下是完整的代码实现:
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int data;
struct ListNode *next;
};
// 读取链表
struct ListNode *readlist() {
int n;
scanf("%d", &n);
struct ListNode *head = NULL, *tail = NULL;
while (n--) {
struct ListNode *node = (struct ListNode *)malloc(sizeof(struct ListNode));
scanf("%d", &(node->data));
node->next = NULL;
if (!head) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
// 删除值为 m 的节点
struct ListNode *deletem(struct ListNode *L, int m) {
struct ListNode *dummy = (struct ListNode *)malloc(sizeof(struct ListNode));
dummy->next = L;
struct ListNode *prev = dummy, *curr = L;
while (curr) {
if (curr->data == m) {
prev->next = curr->next;
free(curr);
curr = prev->next;
} else {
prev = curr;
curr = curr->next;
}
}
L = dummy->next;
free(dummy);
return L;
}
// 打印链表
void printlist(struct ListNode *L) {
struct ListNode *p = L;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int m;
struct ListNode *L = readlist();
scanf("%d", &m);
L = deletem(L, m);
printlist(L);
return 0;
}
函数 `readlist()` 用于读取链表,首先读入链表节点数 `n`,然后循环读入每个节点的值,将其插入链表尾部。函数 `deletem()` 用于删除链表中值为 `m` 的节点,采用双指针法,遍历链表,若当前节点值为 `m`,则删除当前节点,否则继续遍历,直到链表末尾。需要注意的是,为了方便删除链表头节点,可以设置一个哑节点 `dummy`,它的 `next` 指向链表头节点,这样就可以通过 `prev` 指针来删除当前节点。最后,函数 `printlist()` 用于打印链表。
相关推荐
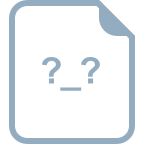
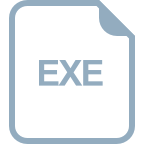
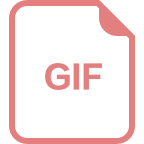














