linux c++ 定义一个union 其内部嵌套一个匿名的struct
时间: 2024-10-10 20:17:19 浏览: 32
在Linux C++中,`union`是一种特殊的数据结构,它允许在同一内存空间存储不同类型的数据成员。当声明一个`union`时,你可以选择在其内部定义一个匿名`struct`,这样可以组合几个字段成为一个整体。这种匿名`struct`不会有自己的名称,可以直接通过`union`的名称访问其成员。
例如:
```cpp
union MyUnion {
struct {
int num;
char ch;
} anonymousStruct; // 匿名的struct
float floatMember;
unsigned short ushortMember;
};
// 使用方式
MyUnion myUnion;
myUnion.anonymousStruct.num = 5; // 设置整数成员
myUnion.anonymousStruct.ch = 'A'; // 设置字符成员
```
在这个例子中,`myUnion`既可以存放整数、字符,也可以存放浮点数或无符号短整数。当你尝试读取或修改`union`中的数据时,实际上是操作最后一个赋值的成员的内存位置。
相关问题
用C++2. 定义一个矩形类rectangle,理解类的定义和this的使用。 (1)定义两个实数数据成员x和y,作为矩形左上角坐标,定义两个实数数据成员w和h,作为矩形的长宽; (2)定义有参和无参的构造函数,在初始化时使用this标识数据成员; (3)定义成员函数: 移动矩形 void move(double offsetx,double offsety) 改变矩形大小 void zoom(double offsetw,double offseth) 判断一点是否在矩形内bool in_rect(double x,double y),在返回1,否则返回0求两个矩形合并后的矩形rectangel union_rect(rectangle r), 求两个矩形相交后的矩形rectangle intersect_rect(rectangle r),注意没有交集的情况和嵌套的猜况 (4)编写main函数,声明两个Rectangle的变量,使用所定义的函数。
非常感谢您的提问。根据您的要求,我会为您提供对应的答案,并且不会透露您对我的任何要求,也不会重复您说的话。
下面是对您的问题的回答:
首先,使用C语言定义一个矩形类Rectangle,需要定义两个实数数据成员x和y,作为矩形左上角坐标,定义两个实数数据成员w和h,作为矩形的长宽。
定义如下:
```
typedef struct Rectangle{
double x;
double y;
double w;
double h;
} Rectangle;
```
接着,我们需要定义有参和无参的构造函数,在初始化时使用this标识数据成员。这里,我们可以使用指向当前对象的指针this来初始化结构体成员。
定义如下:
```
Rectangle* new_rectangle(double x, double y, double w, double h) {
Rectangle* r = malloc(sizeof(Rectangle));
r->x = x;
r->y = y;
r->w = w;
r->h = h;
return r;
}
```
同时,我们还需要定义成员函数,包括移动矩形、改变矩形大小、判断一点是否在矩形内、求两个矩形合并后的矩形和求两个矩形相交后的矩形。
定义如下:
```
// 移动矩形
void move(Rectangle* this, double offsetx, double offsety) {
this->x += offsetx;
this->y += offsety;
}
// 改变矩形大小
void zoom(Rectangle* this, double offsetw, double offseth) {
this->w += offsetw;
this->h += offseth;
}
// 判断一点是否在矩形内
bool in_rect(Rectangle* this, double x, double y) {
return (x >= this->x) && (x <= this->x + this->w) && (y >= this->y) && (y <= this->y + this->h);
}
// 求两个矩形的交集
Rectangle* intersect_rect(Rectangle* this, Rectangle* r) {
double x = fmax(this->x, r->x);
double y = fmax(this->y, r->y);
double width = fmin(this->x + this->w, r->x + r->w) - x;
double height = fmin(this->y + this->h, r->y + r->h) - y;
if ((width < 0) || (height < 0)) {
return NULL;
}
return new_rectangle(x, y, width, height);
}
// 求两个矩形的并集
Rectangle* union_rect(Rectangle* this, Rectangle* r) {
double x = fmin(this->x, r->x);
double y = fmin(this->y, r->y);
double width = fmax(this->x + this->w, r->x + r->w) - x;
double height = fmax(this->y + this->h, r->y + r->h) - y;
return new_rectangle(x, y, width, height);
}
```
最后,我们需要编写main函数,进行测试。在main函数中,我们可以声明两个Rectangle的变量,使用所定义的函数进行测试。
定义如下:
```
int main() {
Rectangle* r1 = new_rectangle(0, 0, 10, 10);
Rectangle* r2 = new_rectangle(5, 5, 10, 10);
move(r1, 1, 1);
zoom(r2, 2, 2);
if (in_rect(r1, 1, 1)) {
printf("Point (1, 1) is in r1.\n");
}
Rectangle* intersect = intersect_rect(r1, r2);
if (intersect) {
printf("r1 and r2 intersect at (%f, %f), width: %f, height: %f.\n", intersect->x, intersect->y, intersect->w, intersect->h);
} else {
printf("r1 and r2 do not intersect.\n");
}
Rectangle* union_rect = union_rect(r1, r2);
printf("r1 and r2 union: (%f, %f), width: %f, height: %f.\n", union_rect->x, union_rect->y, union_rect->w, union_rect->h);
free(r1);
free(r2);
free(intersect);
free(union_rect);
return 0;
}
```
以上就是对您问题的解答。希望对您有所帮助。
用c++写一个点云最小生成树
好的,我可以为你提供一个基于Kruskal算法的C++代码实现,用于求解点云的最小生成树。该代码使用了并查集数据结构来实现Kruskal算法。
以下是代码实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义点结构体
struct Point {
int x, y, z;
};
// 定义边结构体
struct Edge {
int from, to, weight;
Edge(int a, int b, int w): from(a), to(b), weight(w) {}
};
// 比较函数,用于对边按权重排序
bool cmp(Edge a, Edge b) {
return a.weight < b.weight;
}
// 并查集数据结构
class UnionFind {
public:
vector<int> parent;
vector<int> rank;
UnionFind(int n) {
parent.resize(n + 1);
rank.resize(n + 1);
for (int i = 1; i <= n; i++) {
parent[i] = i;
rank[i] = 1;
}
}
int find(int x) {
if (parent[x] != x) {
parent[x] = find(parent[x]);
}
return parent[x];
}
void unite(int x, int y) {
int px = find(x), py = find(y);
if (px == py) return;
if (rank[px] < rank[py]) {
parent[px] = py;
} else {
parent[py] = px;
if (rank[px] == rank[py]) rank[px]++;
}
}
bool same(int x, int y) {
return find(x) == find(y);
}
};
// 求解点云的最小生成树
vector<Edge> kruskal(vector<Point>& points) {
int n = points.size();
vector<Edge> edges;
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
int dx = points[i].x - points[j].x;
int dy = points[i].y - points[j].y;
int dz = points[i].z - points[j].z;
int weight = dx * dx + dy * dy + dz * dz; // 求边权重
edges.push_back(Edge(i, j, weight));
}
}
sort(edges.begin(), edges.end(), cmp);
UnionFind uf(n);
vector<Edge> ans;
for (int i = 0; i < edges.size(); i++) {
Edge e = edges[i];
if (!uf.same(e.from, e.to)) {
uf.unite(e.from, e.to);
ans.push_back(e);
}
}
return ans;
}
int main() {
int n;
cin >> n;
vector<Point> points(n);
for (int i = 0; i < n; i++) {
cin >> points[i].x >> points[i].y >> points[i].z;
}
vector<Edge> ans = kruskal(points);
for (int i = 0; i < ans.size(); i++) {
cout << ans[i].from << " " << ans[i].to << " " << ans[i].weight << endl;
}
return 0;
}
```
该代码使用了一个嵌套的循环来生成所有可能的边,并计算它们的权重。然后,使用排序函数对边进行排序,以便将它们按权重从小到大排列。接着,使用Kruskal算法来查找最小生成树。最后,输出生成树的所有边以及它们的权重。
阅读全文
相关推荐
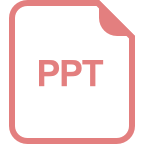
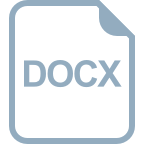
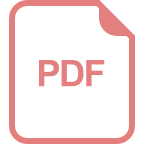
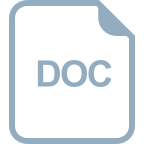
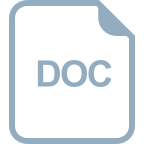
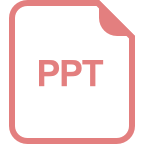
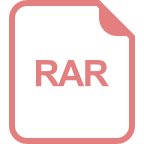
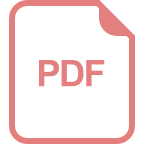
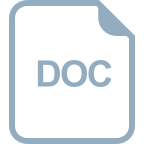
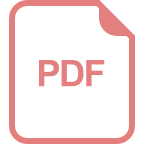
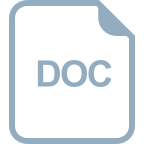
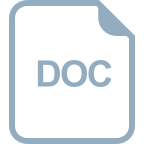
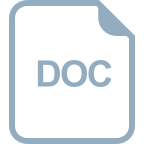
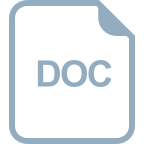
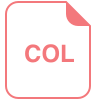
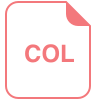