用c语言编写程序完成输出二叉树中值为x的结点的所有祖先结点。假设值为x的结点不超过一个
时间: 2024-10-16 13:17:36 浏览: 33
在C语言中,为了找到二叉树中值为x的节点的所有祖先节点,我们可以采用深度优先搜索(Depth-First Search, DFS)的方式,结合递归技术。首先,我们需要定义一个结构体表示二叉树的节点,包含值(val)、左子节点(left)和右子节点(right)。然后,我们可以在DFS函数中处理这个问题:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 深度优先搜索,寻找祖先节点
void findAncestors(TreeNode* root, TreeNode* x, TreeNode** ancestors) {
if (!root || root->val == x->val)
return;
// 如果当前节点不是目标值,添加到祖先链表的头部
if (ancestors)
ancestors = &(*ancestors)->next;
*ancestors = (TreeNode*)malloc(sizeof(TreeNode));
(*ancestors)->val = root->val;
(*ancestors)->left = NULL;
(*ancestors)->right = NULL;
// 递归检查左右子节点
findAncestors(root->left, x, ancestors);
findAncestors(root->right, x, ancestors);
}
int main() {
// 创建示例二叉树
TreeNode* root = malloc(sizeof(TreeNode));
root->val = 5;
root->left = malloc(sizeof(TreeNode));
root->left->val = 3;
root->left->left = malloc(sizeof(TreeNode));
root->left->left->val = 1;
root->left->right = malloc(sizeof(TreeNode));
root->left->right->val = 4;
root->right = malloc(sizeof(TreeNode));
root->right->val = 6;
root->right->left = malloc(sizeof(TreeNode));
root->right->left->val = 7;
root->right->right = malloc(sizeof(TreeNode));
root->right->right->val = 8;
TreeNode* targetNode = ...; // 假设已经找到了值为x的节点
TreeNode* ancestors = NULL;
findAncestors(root, targetNode, &ancestors);
printf("Value %d 的所有祖先节点:", targetNode->val);
while (ancestors != NULL) {
printf("%d ", ancestors->val);
ancestors = ancestors->next;
}
printf("\n");
free(ancestors); // 释放内存
return 0;
}
```
在这个例子中,`findAncestors` 函数会返回从根节点到给定节点的路径上所有祖先节点构成的链表。如果没有找到目标值,链表将为空。
阅读全文
相关推荐
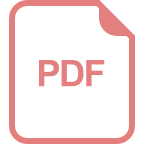
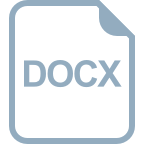
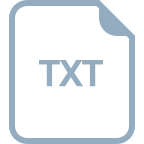















