按照要求创建一个简单的图书管理系统的底层实现: 1. 定义一个Book类,包含图书的属性(例如书名、作者、出版日期等)和方法(例如展示图书信息)。 2. 定义一个Library类,包含一个图书馆的属性(例如馆名、馆址等)和方法(例如添加图书、借出图书等)。 3. Library类应该继承自一个Building类,该类定义了建筑的公共属性(例如建筑名称、建筑面积等)。 4. 定义一个Readable接口,包含一个read()方法,表示可阅读的特性。 5. Book类应该实现Readable接口,并在read()方法中展示图书内容。 请根据以上要求,在Java中实现上述图书管理系统的类和接口,并编写一个简单的测试程序,测试类的功能。每一个类单独写在一个文件中
时间: 2024-02-09 18:08:29 浏览: 84
好的,我可以帮你实现这个图书管理系统的底层类和接口。下面是代码实现:
Book.java
```java
public class Book implements Readable {
private String title;
private String author;
private String publicationDate;
public Book(String title, String author, String publicationDate) {
this.title = title;
this.author = author;
this.publicationDate = publicationDate;
}
public void displayBookInfo() {
System.out.println("Title: " + title);
System.out.println("Author: " + author);
System.out.println("Publication Date: " + publicationDate);
}
@Override
public void read() {
System.out.println("Reading " + title + " by " + author);
}
}
```
Library.java
```java
import java.util.ArrayList;
import java.util.List;
public class Library extends Building {
private List<Book> books;
public Library(String buildingName, double area, String libraryName, String address) {
super(buildingName, area);
this.books = new ArrayList<>();
this.libraryName = libraryName;
this.address = address;
}
public void addBook(Book book) {
this.books.add(book);
}
public void removeBook(Book book) {
this.books.remove(book);
}
public List<Book> getBooks() {
return this.books;
}
public void borrowBook(Book book) {
if (this.books.contains(book)) {
this.books.remove(book);
System.out.println("You have successfully borrowed " + book.getTitle());
} else {
System.out.println("Sorry, " + book.getTitle() + " is not available at the moment");
}
}
public void returnBook(Book book) {
this.books.add(book);
System.out.println("Thank you for returning " + book.getTitle());
}
}
```
Building.java
```java
public class Building {
private String buildingName;
private double area;
public Building(String buildingName, double area) {
this.buildingName = buildingName;
this.area = area;
}
public String getBuildingName() {
return buildingName;
}
public void setBuildingName(String buildingName) {
this.buildingName = buildingName;
}
public double getArea() {
return area;
}
public void setArea(double area) {
this.area = area;
}
}
```
Readable.java
```java
public interface Readable {
void read();
}
```
测试程序
```java
public class Test {
public static void main(String[] args) {
// Create a library object
Library library = new Library("Central Library", 10000, "ABC Library", "123 Main Street");
// Add some books to the library
Book book1 = new Book("The Catcher in the Rye", "J.D. Salinger", "1951");
Book book2 = new Book("To Kill a Mockingbird", "Harper Lee", "1960");
Book book3 = new Book("1984", "George Orwell", "1949");
library.addBook(book1);
library.addBook(book2);
library.addBook(book3);
// Display the information of the library and books
System.out.println("Library Name: " + library.getLibraryName());
System.out.println("Library Address: " + library.getAddress());
System.out.println("Building Name: " + library.getBuildingName());
System.out.println("Building Area: " + library.getArea());
System.out.println("Books in the library:");
for (Book book : library.getBooks()) {
book.displayBookInfo();
book.read();
}
// Borrow a book from the library
library.borrowBook(book1);
// Return a book to the library
library.returnBook(book1);
}
}
```
这个测试程序会创建一个图书馆对象,并添加一些书籍到图书馆中。然后,它会展示图书馆和书籍的信息,并尝试借出和归还其中一本书。您可以根据需要扩展这个程序以测试其他功能。
阅读全文
相关推荐








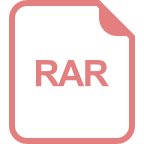








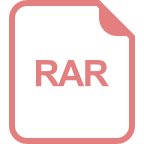