python气象画时间序列图
时间: 2023-08-29 18:05:31 浏览: 187
您可以使用Python中的matplotlib库来绘制时间序列图。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
import pandas as pd
# 读取数据
data = pd.read_csv('your_data.csv')
# 将日期转换为datetime格式
data['date'] = pd.to_datetime(data['date'])
# 设置x轴为日期
plt.gca().xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.gca().xaxis.set_major_locator(mdates.DayLocator())
# 绘图
plt.plot(data['date'], data['temperature'])
# 添加标签和标题
plt.xlabel('Date')
plt.ylabel('Temperature (Celsius)')
plt.title('Temperature Time Series')
# 显示图像
plt.show()
```
您需要将“your_data.csv”替换为您的实际数据文件名,并确保数据文件包含“date”和“temperature”两列。此代码将显示温度随时间变化的时间序列图。您可以根据需要进行修改和自定义。
相关问题
python 气象画图
您可以使用 Python 的 Matplotlib 库来绘制气象图。Matplotlib 提供了多种绘图函数和工具,可以绘制线图、散点图、柱状图、等高线图、3D 图等。对于气象数据,可以使用 Matplotlib 绘制温度、湿度、气压等变量的时间序列图或空间分布图。
以下是一个简单的示例代码,用于绘制温度时间序列图:
```python
import matplotlib.pyplot as plt
import pandas as pd
# 读取气象数据
data = pd.read_csv('weather.csv')
# 绘制温度时间序列图
plt.plot(data['date'], data['temperature'])
plt.xlabel('Date')
plt.ylabel('Temperature (°C)')
plt.title('Temperature Time Series')
plt.show()
```
其中,`weather.csv` 是包含日期和温度数据的 CSV 文件。您需要根据实际情况修改文件名和变量名。
python气象观测
Python可以用于气象观测数据的处理和分析。以下是一些常见的Python库和工具,可用于气象观测相关的任务:
1. NumPy:用于处理数值数据和数组操作的基础库。
2. Pandas:用于处理和分析数据的库,可以方便地读取、处理和操作气象观测数据。
3. Matplotlib:用于绘制各种图表和数据可视化的库,可以绘制气象观测数据的时间序列、散点图、柱状图等。
4. SciPy:提供了一些科学计算和统计分析的函数,可用于气象数据的处理和分析。
5. Basemap:一个Python地图绘制工具,可用于绘制气象观测数据在地理空间上的分布。
6. MetPy:专门用于气象数据处理和分析的库,提供了许多用于处理气象观测数据的功能。
7. xarray:一个用于处理多维数组数据的库,特别适用于气象观测数据的处理和分析。
8. Cartopy:用于绘制地图和地理数据可视化的库,可用于展示气象观测数据在地理空间上的分布。
使用这些工具,你可以读取、处理、分析和可视化气象观测数据,并进行相关的统计分析和模型建立。
阅读全文
相关推荐
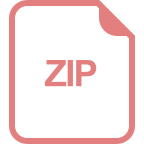
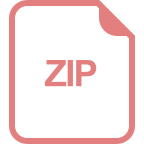
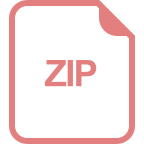
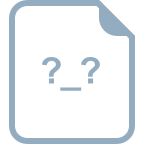
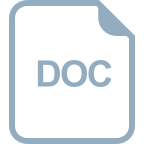
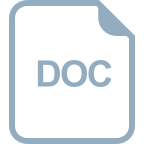
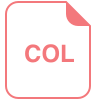
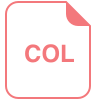
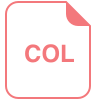
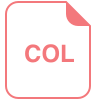
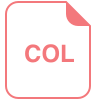
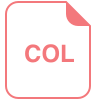
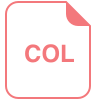