写一段循环双向链表的代码
时间: 2024-03-04 20:44:01 浏览: 121
下面是一个循环双向链表的代码实现:
```
class Node:
def __init__(self, val=None, prev=None, next=None):
self.val = val
self.prev = prev
self.next = next
class DoublyLinkedList:
def __init__(self):
self.head = Node()
self.tail = Node()
self.head.next = self.tail
self.tail.prev = self.head
def add_front(self, val):
node = Node(val, self.head, self.head.next)
self.head.next.prev = node
self.head.next = node
def add_back(self, val):
node = Node(val, self.tail.prev, self.tail)
self.tail.prev.next = node
self.tail.prev = node
def remove_front(self):
if self.head.next == self.tail:
return None
node = self.head.next
self.head.next = node.next
node.next.prev = self.head
return node.val
def remove_back(self):
if self.tail.prev == self.head:
return None
node = self.tail.prev
self.tail.prev = node.prev
node.prev.next = self.tail
return node.val
def __str__(self):
vals = []
node = self.head.next
while node != self.tail:
vals.append(str(node.val))
node = node.next
return " <-> ".join(vals)
```
这个实现包括了添加元素到头尾、删除头尾元素、以及打印链表的功能。需要注意的是,在初始化时需要创建两个哨兵节点,一个作为头节点,一个作为尾节点,并将它们互相连接起来,这样就形成了一个循环链表。同时,添加元素时需要注意更新前后节点的指针。
阅读全文
相关推荐

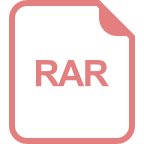












