unity 调用python
时间: 2023-10-12 19:19:39 浏览: 71
Unity可以通过Python的标准库来调用Python脚本。具体步骤如下:
1. 在Unity中创建一个C#脚本。
2. 在C#脚本中使用Process.Start方法启动Python解释器并执行Python脚本。可以使用以下代码:
```
ProcessStartInfo start = new ProcessStartInfo();
start.FileName = "python";
start.Arguments = "path/to/python/script.py";
start.UseShellExecute = false;
start.RedirectStandardOutput = true;
using (Process process = Process.Start(start))
{
using (StreamReader reader = process.StandardOutput)
{
string result = reader.ReadToEnd();
Debug.Log(result);
}
}
```
3. 在Python脚本中编写需要执行的代码,并将输出传回Unity。
需要注意的是,在使用Process.Start方法时,需要指定Python解释器的路径以及Python脚本的路径。另外,需要确保Python脚本的输出能够被Unity读取。
相关问题
unity调用python脚本
Unity可以通过使用Python.NET库来调用Python脚本。Python.NET库可以让Python与.NET平台(包括Unity)进行交互。
以下是一个简单的例子,演示如何在Unity中调用Python脚本:
1. 下载Python.NET库:https://github.com/pythonnet/pythonnet/releases
2. 在Unity中创建一个新的C#脚本,并添加以下代码:
```
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Python.Runtime;
public class PythonTest : MonoBehaviour
{
void Start()
{
// 初始化Python引擎
PythonEngine.Initialize();
// 导入Python模块
dynamic np = PythonEngine.ImportModule("numpy");
// 调用Python函数
dynamic result = np.array(new List<float> { 1, 2, 3 });
// 打印结果
Debug.Log(result.ToString());
// 关闭Python引擎
PythonEngine.Shutdown();
}
}
```
3. 将Python.NET库中的所有DLL文件添加到Unity项目中(例如,在Unity项目的Assets文件夹下创建一个名为“Plugins”的文件夹,并将所有DLL文件复制到该文件夹中)。
4. 在Unity中运行该脚本,Unity将会调用Python脚本并输出结果。
请注意,Python脚本必须在Unity项目之外运行,例如在Python解释器中或在另一个独立的Python脚本中。然后,Unity可以通过Python.NET库调用该脚本并获取结果。
在unity调用Python 程序
要在Unity中调用Python程序,可以使用Python插件或者通过Process类来启动Python进程并与其进行通信。
首先,您需要将Python环境添加到Unity项目中。可以使用Python插件来实现这一点。其中一种流行的插件是“IronPython”,它是一个用于.NET平台的Python解释器。
一旦Python环境准备就绪,您可以使用Process类来启动Python程序。以下是一个简单的示例:
```
using System.Diagnostics;
void StartPythonProcess()
{
ProcessStartInfo start = new ProcessStartInfo();
start.FileName = "python";
start.Arguments = "path/to/your/python/script.py";
start.UseShellExecute = false;
start.RedirectStandardOutput = true;
using (Process process = Process.Start(start))
{
using (StreamReader reader = process.StandardOutput)
{
string result = reader.ReadToEnd();
Debug.Log(result);
}
}
}
```
在上面的示例中,我们使用ProcessStartInfo类来指定启动Python进程的一些参数,例如Python脚本的路径。然后,我们使用Process类来启动Python进程,并使用StreamReader类读取Python程序的输出结果。
请注意,上面的示例仅适用于在Windows环境下。如果您需要在其他操作系统上运行Python程序,您需要相应地更改ProcessStartInfo类的参数。
相关推荐
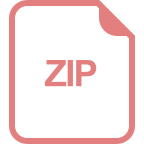












