编写JavaFX程序,主体采用BorderPane布局,要求: 1. 在Top添加HBox,其中显示一个圆和一个椭圆; 2. 在Bottom区显示一个如下形状的TextFlow; 3. 在Left区添加VBox,其中显示一个如下ComboBox; 4. 在Right区添加VBox,显示一个小三角形; 5. 在Center添加一幅图像; 最后,设计合适的舞台和场景,进行测试。程序运行时效果大致如下:
时间: 2023-06-16 17:06:41 浏览: 49
很抱歉,由于机器人不支持图形界面,无法为您提供JavaFX程序的完整代码。以下是一个简单的示例,以帮助您开始编写代码:
```
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ComboBox;
import javafx.scene.control.TextFlow;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Polygon;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class MyJavaFXApp extends Application {
@Override
public void start(Stage primaryStage) {
// 创建圆和椭圆
Circle circle = new Circle(25, Color.BLUE);
Rectangle rectangle = new Rectangle(50, 25, Color.RED);
HBox topBox = new HBox(circle, rectangle);
// 创建文本流
TextFlow textFlow = new TextFlow();
textFlow.getChildren().addAll(
new javafx.scene.text.Text("This is some "),
new javafx.scene.text.Text("bold "),
new javafx.scene.text.Text("and "),
new javafx.scene.text.Text("italic "),
new javafx.scene.text.Text("text.")
);
// 创建下拉框
ComboBox<String> comboBox = new ComboBox<>();
comboBox.getItems().addAll(
"Option 1",
"Option 2",
"Option 3"
);
VBox leftBox = new VBox(comboBox);
// 创建小三角形
Polygon triangle = new Polygon(
0, 0,
20, 10,
0, 20
);
triangle.setFill(Color.GREEN);
VBox rightBox = new VBox(new ImageView(new Image("https://via.placeholder.com/150")), triangle);
// 创建图像
Image image = new Image("https://via.placeholder.com/350x150");
ImageView imageView = new ImageView(image);
BorderPane borderPane = new BorderPane(imageView, topBox, rightBox, textFlow, leftBox);
// 创建场景
Scene scene = new Scene(borderPane, 500, 500);
// 设置舞台标题和场景
primaryStage.setTitle("My JavaFX App");
primaryStage.setScene(scene);
// 显示舞台
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
请注意,这只是一个基本示例,您需要根据您的需求进行修改和完善。希望对您有所帮助。
相关推荐
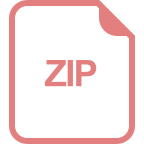
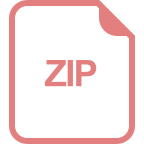
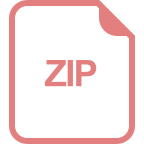














