编写JavaFX程序,主体采用BorderPane布局,要求: 1. 在Top添加HBox,其中显示一个圆和一个椭圆; 2. 在Bottom区显示一个如下形状的TextFlow;
时间: 2024-06-09 10:04:22 浏览: 56
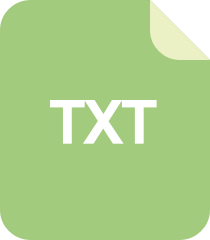
一个简单的Java布局的程序
以下是一个示例JavaFX程序,实现了要求的布局和显示效果:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Ellipse;
import javafx.scene.text.Text;
import javafx.scene.text.TextFlow;
import javafx.stage.Stage;
public class BorderPaneExample extends Application {
@Override
public void start(Stage primaryStage) {
// 创建圆和椭圆
Circle circle = new Circle(30, Color.RED);
Ellipse ellipse = new Ellipse(40, 20, Color.BLUE);
// 创建HBox,添加圆和椭圆
HBox hbox = new HBox(10, circle, ellipse);
hbox.setPadding(new Insets(10));
// 创建TextFlow,添加多个Text
TextFlow textFlow = new TextFlow();
textFlow.setPadding(new Insets(10));
textFlow.setStyle("-fx-background-color: gray;");
Text text1 = new Text("Hello ");
text1.setStyle("-fx-font-weight: bold;");
Text text2 = new Text("world!");
text2.setFill(Color.RED);
Text text3 = new Text(" This is a ");
Text text4 = new Text("TextFlow");
text4.setFill(Color.GREEN);
textFlow.getChildren().addAll(text1, text2, text3, text4);
// 创建BorderPane,添加HBox和TextFlow
BorderPane borderPane = new BorderPane();
borderPane.setTop(hbox);
borderPane.setBottom(textFlow);
// 创建Scene并设置到Stage
Scene scene = new Scene(borderPane, 400, 300);
primaryStage.setScene(scene);
primaryStage.setTitle("BorderPane Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
运行程序后,可以看到如下效果:

阅读全文
相关推荐
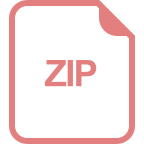

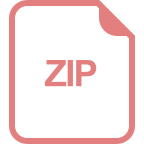
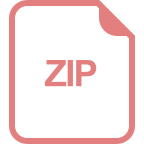
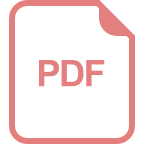
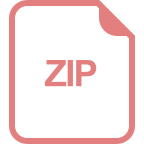
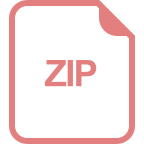
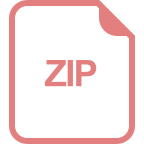
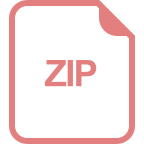
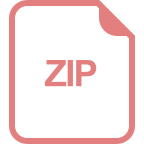
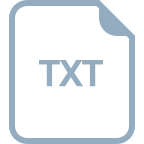
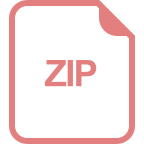
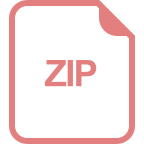
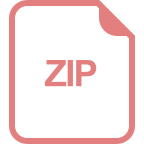
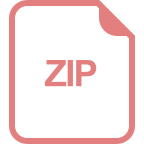
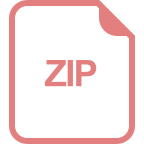