java 实现判断点在图形内
时间: 2024-06-09 18:09:14 浏览: 71
判断一个点是否在一个图形内,需要先确定这个图形的类型。以下是一些常见图形的判断方法:
1. 矩形:给定一个矩形的左上角和右下角的两个点,判断一个点是否在这个矩形内部,只需要判断该点的 x 坐标是否在矩形的左右边界之间,y 坐标是否在矩形的上下边界之间即可。
2. 圆形:给定一个圆心坐标和半径,判断一个点是否在圆内,只需要计算该点到圆心的距离是否小于半径。
3. 多边形:给定多个点的坐标,组成一个多边形,判断一个点是否在多边形内,可以使用射线法、顶点法或者向量法等算法。
以下是使用射线法判断点是否在任意多边形内的 Java 代码示例:
```
public class PointInPolygon {
public static boolean isInside(Point2D.Double[] polygon, Point2D.Double point) {
int intersections = 0;
for (int i = 0; i < polygon.length; i++) {
Point2D.Double a = polygon[i];
Point2D.Double b = polygon[(i + 1) % polygon.length];
if (a.y == b.y) {
continue;
}
if (point.y < Math.min(a.y, b.y) || point.y >= Math.max(a.y, b.y)) {
continue;
}
double x = (point.y - a.y) * (b.x - a.x) / (b.y - a.y) + a.x;
if (x > point.x) {
intersections++;
}
}
return intersections % 2 != 0;
}
public static void main(String[] args) {
Point2D.Double[] polygon = new Point2D.Double[] {
new Point2D.Double(0, 0),
new Point2D.Double(0, 4),
new Point2D.Double(4, 4),
new Point2D.Double(4, 0)
};
Point2D.Double point = new Point2D.Double(2, 2);
if (isInside(polygon, point)) {
System.out.println("Point is inside the polygon");
} else {
System.out.println("Point is outside the polygon");
}
}
}
```
这段代码中,`isInside` 方法接收一个点数组和一个点对象,判断该点是否在指定的多边形内。该方法使用射线法,对于多边形中的每条边,如果该边与射线相交,则将交点个数加 1。最后,如果交点个数为奇数,则该点在多边形内,否则在多边形外。
阅读全文
相关推荐


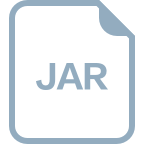
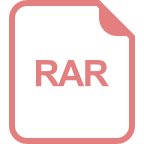





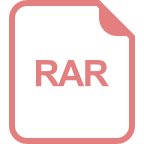

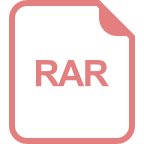





