输出一段java贪吃蛇代码
时间: 2023-12-23 07:51:13 浏览: 72
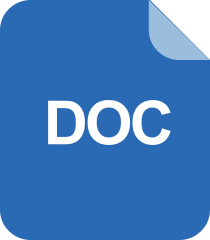
贪吃蛇java代码
当然可以!以下是简单的Java贪吃蛇代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Snake extends JPanel implements Runnable, KeyListener {
private Thread thread;
private boolean running;
private int score;
private int[] snakeX = new int[700];
private int[] snakeY = new int[700];
private int lengthOfSnake = 3;
private int appleX;
private int appleY;
private boolean leftDirection = false;
private boolean rightDirection = true;
private boolean upDirection = false;
private boolean downDirection = false;
public Snake() {
addKeyListener(this);
setFocusable(true);
setPreferredSize(new Dimension(500,500));
initGame();
}
public void initGame() {
running = true;
score = 0;
lengthOfSnake = 3;
leftDirection = false;
rightDirection = true;
upDirection = false;
downDirection = false;
for (int i = 0; i < lengthOfSnake; i++) {
snakeX[i] = 50 - i*10;
snakeY[i] = 50;
}
newApple();
if (thread == null) {
thread = new Thread(this);
thread.start();
}
}
public void newApple() {
appleX = (int) (Math.random() * 50) * 10;
appleY = (int) (Math.random() * 50) * 10;
}
protected void paintComponent(Graphics graphics) {
super.paintComponent(graphics);
draw(graphics);
}
public void draw(Graphics graphics) {
if (running) {
graphics.setColor(Color.red);
graphics.fillOval(appleX, appleY, 10, 10);
for (int i = 0; i < lengthOfSnake; i++) {
if (i == 0) {
graphics.setColor(Color.GRAY);
graphics.fillRect(snakeX[i], snakeY[i], 10, 10);
}
else {
graphics.setColor(Color.darkGray);
graphics.fillRect(snakeX[i], snakeY[i], 10, 10);
}
}
graphics.setColor(Color.WHITE);
graphics.drawString("Score: "+ score, 10, 20);
} else {
gameOver(graphics);
}
}
public void move() {
for (int i = lengthOfSnake; i > 0 ; i--) {
snakeX[i] = snakeX[i-1];
snakeY[i] = snakeY[i-1];
}
if (leftDirection) {
snakeX[0] -= 10;
}
if (rightDirection) {
snakeX[0] += 10;
}
if (upDirection) {
snakeY[0] -= 10;
}
if (downDirection) {
snakeY[0] += 10;
}
}
public void checkApple() {
if (snakeX[0] == appleX && snakeY[0] == appleY) {
lengthOfSnake++;
score++;
newApple();
}
}
public void checkCollisions() {
for (int i = lengthOfSnake; i > 0 ; i--) {
if (i > 4 && snakeX[0] == snakeX[i] && snakeY[0] == snakeY[i]) {
running = false;
}
}
if (snakeX[0] < 0 || snakeX[0] > 490 || snakeY[0] < 0 || snakeY[0] > 490) {
running = false;
}
if (!running) {
thread = null;
}
}
public void gameOver(Graphics graphics) {
String message = "Game Over";
Font font = new Font("Times New Roman", Font.BOLD, 30);
FontMetrics metrics = getFontMetrics(font);
graphics.setColor(Color.WHITE);
graphics.setFont(font);
graphics.drawString(message, (500 - metrics.stringWidth(message)) / 2, 250);
}
public void run() {
while (running) {
move();
checkApple();
checkCollisions();
repaint();
try {
Thread.sleep(60);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if ((key == KeyEvent.VK_LEFT) && (!rightDirection)) {
leftDirection = true;
upDirection = false;
downDirection = false;
}
if ((key == KeyEvent.VK_RIGHT) && (!leftDirection)) {
rightDirection = true;
upDirection = false;
downDirection = false;
}
if ((key == KeyEvent.VK_UP) && (!downDirection)) {
upDirection = true;
rightDirection = false;
leftDirection = false;
}
if ((key == KeyEvent.VK_DOWN) && (!upDirection)) {
downDirection = true;
rightDirection = false;
leftDirection = false;
}
}
public void keyTyped(KeyEvent e) {}
public void keyReleased(KeyEvent e) {}
public static void main(String[] args) {
JFrame frame = new JFrame("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(new Snake(), BorderLayout.CENTER);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
带有图形界面,可以通过方向键控制蛇的移动,得分与死亡判定等功能。
阅读全文
相关推荐
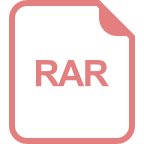
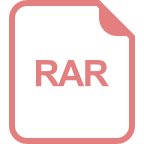
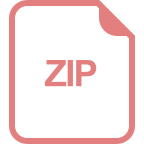
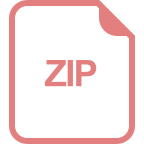
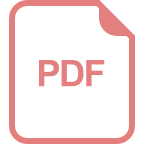
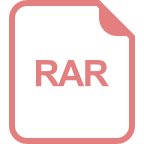
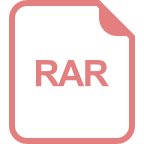
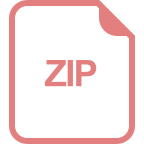
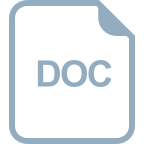
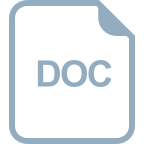
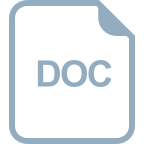
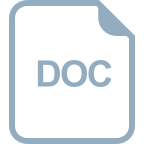
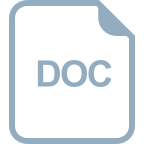
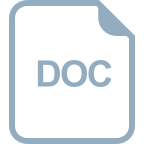
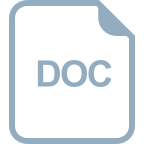
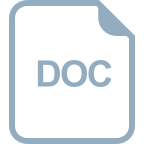
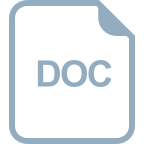