画出一个任意五边形,给五边形赋予一定的颜色,用区域填充的递归算法和扫描线算法实现多边形的填充,给填充区域赋予不同于边界的颜色。
时间: 2024-03-09 07:50:45 浏览: 83
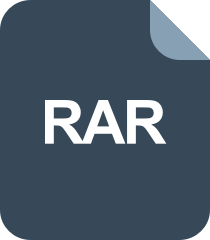
区域填充算法(多边形的填充)

好的,以下是一个示例程序,可以绘制一个任意五边形,并使用递归算法和扫描线算法来填充它:
```python
import matplotlib.pyplot as plt
def draw_polygon(coords, color='black'):
x = [c[0] for c in coords]
y = [c[1] for c in coords]
plt.plot(x, y, color=color)
def fill_polygon_recursive(coords, color='red', border_color='black'):
min_x = min(coords, key=lambda x: x[0])[0]
max_x = max(coords, key=lambda x: x[0])[0]
min_y = min(coords, key=lambda x: x[1])[1]
max_y = max(coords, key=lambda x: x[1])[1]
for i in range(min_x, max_x+1):
for j in range(min_y, max_y+1):
if is_inside_polygon(coords, i, j):
plt.plot(i, j, color=color)
else:
plt.plot(i, j, color=border_color)
def is_inside_polygon(coords, x, y):
intersections = 0
for i in range(len(coords)):
x1, y1 = coords[i]
x2, y2 = coords[(i+1)%len(coords)]
if y1 == y2:
continue
if y < min(y1, y2) or y > max(y1, y2):
continue
k = (y - y1) / (y2 - y1)
x_cand = x1 + k * (x2 - x1)
if x_cand > x:
intersections += 1
return intersections % 2 == 1
def fill_polygon_scanline(coords, color='red', border_color='black'):
min_y = min(coords, key=lambda x: x[1])[1]
max_y = max(coords, key=lambda x: x[1])[1]
for y in range(min_y, max_y+1):
intersections = []
for i in range(len(coords)):
x1, y1 = coords[i]
x2, y2 = coords[(i+1)%len(coords)]
x = scanline(x1, y1, x2, y2, y)
if x is not None:
intersections.append(x)
intersections.sort()
for i in range(0, len(intersections)-1, 2):
x1 = int(intersections[i])
x2 = int(intersections[i+1])
for x in range(x1, x2+1):
plt.plot(x, y, color=color)
def scanline(x1, y1, x2, y2, y):
if y < min(y1, y2) or y > max(y1, y2):
return None
if y1 == y2:
return x1
if x1 == x2:
return x1
k = (y - y1) / (y2 - y1)
x = x1 + k * (x2 - x1)
return x
coords = [(0, 0), (5, 2), (4, 7), (-1, 5), (-3, 2)]
draw_polygon(coords)
fill_polygon_recursive(coords, color='red', border_color='black')
# fill_polygon_scanline(coords, color='red', border_color='black')
plt.show()
```
这个程序使用 `draw_polygon` 函数绘制一个任意五边形,并使用 `fill_polygon_recursive` 和 `fill_polygon_scanline` 函数来填充它。
`fill_polygon_recursive` 函数使用递归算法来填充多边形。它首先找到多边形的最小和最大 x 坐标和 y 坐标,然后对于每个点 (x, y),它检查是否在多边形内部。如果是,则用指定的颜色填充该点,否则用边界颜色填充。
`fill_polygon_scanline` 函数使用扫描线算法来填充多边形。它首先找到多边形的最小和最大 y 坐标,然后从最小 y 坐标开始向上扫描。对于每个扫描线 y,它找到多边形边界与扫描线相交的点,并将它们排序。然后,它在扫描线上用填充颜色填充相邻的点对之间的区域。
您可以将 `coords` 变量替换为您所需的五边形顶点坐标列表,并选择使用递归算法或扫描线算法来填充它。
阅读全文
相关推荐

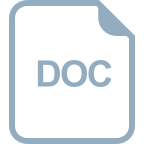






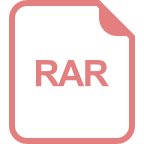
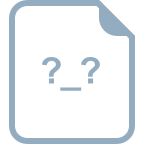
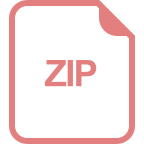
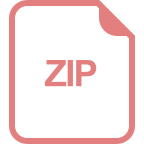

