于条件变量和队列进行生产者-消费者同步,创建队列对象和条件变量对象,创建消费者进程和生产者进程,生产者生产产品放入队列并通过条件变量通知消费者,消费者收到条件变量的通知从队列中消费一个产品。
时间: 2024-10-24 22:09:59 浏览: 17
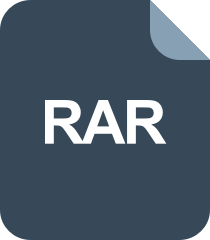
consumer_producer.rar_操作系统_生产 消费_生产者-消费者问题_进程调度
在生产者-消费者模型中,条件变量和队列一起用于解决并发中的同步问题。首先,你需要:
1. **创建资源**:创建一个`Queue`对象作为共享的数据结构,用于存放生产者产生的商品。例如在Python中可以使用`queue.Queue`,在Java中则有`java.util.concurrent.LinkedList`。
2. **条件变量**:这是Java提供的工具,它允许线程在某个条件满足(如队列非空)时进入等待状态,在条件改变时被唤醒。`java.util.concurrent.Condition`是一个与锁关联的对象,通常附属于`Lock`。
3. **生产者进程**:这个进程负责生成商品并将其添加到队列。当队列已满时,它会调用条件变量的`await()`方法进入等待状态;当队列空间可用时,通过`signal()`或`signalAll()`唤醒其他等待的消费者。
4. **消费者进程**:接收条件变量的通知后,从队列中取出商品并处理。如果队列为空,它会调用条件变量的`await()`方法直到商品可用。
这种模式确保了数据的一致性和公平性,避免了死锁,并提高了资源利用效率。以下是简单示例代码(Java):
```java
import java.util.concurrent.*;
public class ProducerConsumer {
private final Queue<Integer> queue = new LinkedList<>();
private final Condition notFull = new Condition(new ReentrantLock());
private final Condition notEmpty = new Condition(new ReentrantLock());
public void producer(int num) throws InterruptedException {
for (int i = 0; i < num; i++) {
synchronized (this) {
while (queue.size() == MAX_SIZE) {
notFull.await();
}
queue.add(i);
notEmpty.signal();
}
}
}
public void consumer() throws InterruptedException {
while (true) {
synchronized (this) {
while (queue.isEmpty()) {
notEmpty.await();
}
int item = queue.poll();
// process the item
System.out.println("Consumed item: " + item);
}
}
}
}
```
阅读全文
相关推荐
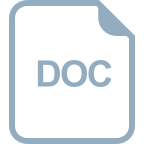
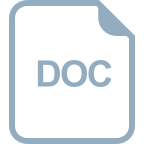
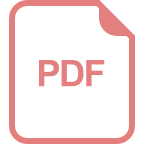
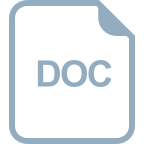
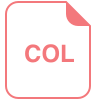
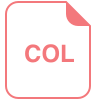
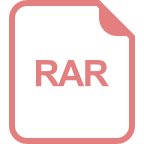
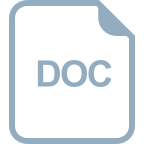
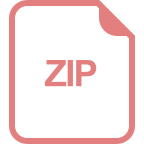
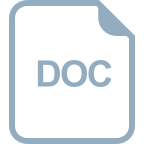
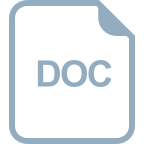
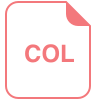





