条件变量 + 队列 实现生产消费者模型 C语言代码
时间: 2024-09-20 08:01:46 浏览: 40
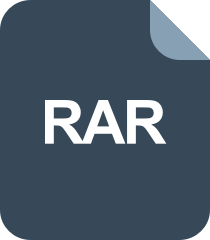
C语言,消费者和生产者模式
在C语言中,可以使用条件变量配合队列(如`pthread_cond_t`和`pthread_mutex_t`等互斥量)来实现生产者消费者模型,这是一种常见的并发控制机制。下面是一个简单的示例:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 10
#define PRODUCER_THREADS 2
#define CONSUMER_THREADS 2
typedef struct {
int queue[MAX_QUEUE_SIZE];
int front, rear;
pthread_cond_t cond;
pthread_mutex_t mutex;
} Queue;
void* producer(void* arg) {
Queue* q = (Queue*)arg;
while (1) {
// 生产数据并判断队列是否满
int data = rand() % MAX_QUEUE_SIZE;
pthread_mutex_lock(&q->mutex);
if (q->rear == q->front) { // 如果队列已满
printf("Producer blocked due to full queue.\n");
pthread_cond_wait(&q->cond, &q->mutex); // 等待唤醒
} else {
q->queue[q->rear] = data; // 入队
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
printf("Produced %d, queue size: %d\n", data, q->rear - q->front);
pthread_cond_signal(&q->cond); // 唤醒消费者
}
pthread_mutex_unlock(&q->mutex);
}
}
void* consumer(void* arg) {
Queue* q = (Queue*)arg;
while (1) {
pthread_mutex_lock(&q->mutex);
if (q->front == q->rear) { // 如果队列为空
printf("Consumer blocked due to empty queue.\n");
pthread_cond_wait(&q->cond, &q->mutex); // 等待唤醒
} else {
int data = q->queue[q->front]; // 出队
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
printf("Consumed %d, queue size: %d\n", data, q->rear - q->front);
pthread_cond_signal(&q->cond); // 唤醒其他消费者或生产者
}
pthread_mutex_unlock(&q->mutex);
}
}
int main() {
Queue q;
for (int i = 0; i < 2; ++i) {
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, &q);
pthread_create(&consumer_thread, NULL, consumer, &q);
}
// 等待所有线程结束
for (int i = 0; i < 2; ++i) {
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
}
return 0;
}
```
在这个例子中,生产者和消费者线程通过条件变量`cond`和锁`mutex`协调操作,生产者入队和消费者出队,当队列满或空时,使用`pthread_cond_wait`让当前线程进入等待状态,直到有其他线程唤醒它。
阅读全文
相关推荐
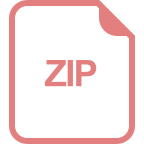
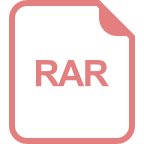















