pycharm爬虫代码
时间: 2023-10-12 08:03:30 浏览: 146
以下是一个简单的 Python 爬虫程序示例,使用 PyCharm 编写:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
```
这个程序会请求指定 URL 的网页并使用 BeautifulSoup 库解析 HTML 内容,然后找到所有链接并打印输出。在 PyCharm 中,你可以使用 Python Console 进行交互式测试和调试。
相关问题
pycharm爬虫代码案例
以下是一个使用 PyCharm 编写的简单爬虫代码案例:
```python
import requests
from bs4 import BeautifulSoup
# 发送 HTTP 请求获取数据
url = 'https://www.example.com'
response = requests.get(url)
# 解析 HTML 数据
soup = BeautifulSoup(response.text, 'html.parser')
links = soup.find_all('a')
# 遍历所有链接并打印
for link in links:
print(link.get('href'))
```
这个代码会向指定的 URL 发送 HTTP 请求,然后使用 BeautifulSoup 库解析 HTML 数据。最后,它会遍历所有的链接并打印它们的地址。在 PyCharm 中运行这个代码非常简单,只需要创建一个 Python 项目,然后将这个代码复制到 main.py 文件中。然后点击运行按钮即可执行代码。
pycharm爬虫代码爬取CSDN
以下是一个使用 requests 和 BeautifulSoup 库爬取 CSDN 首页文章列表的代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 发送请求并获取响应
url = 'https://www.csdn.net/'
response = requests.get(url)
# 使用 BeautifulSoup 解析 HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 获取文章列表
articles = soup.select('.feedlist_mod > .clearfix > dl')
for article in articles:
title = article.select_one('.title > h2 > a').text.strip()
link = article.select_one('.title > h2 > a')['href']
summary = article.select_one('.summary').text.strip()
print(title)
print(link)
print(summary)
```
请注意,CSDN 的使用协议禁止对网站进行爬取,请遵守相关规定。
相关推荐
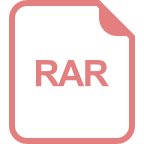










