package Shoot; import java.awt.Graphics; @SuppressWarnings("unused") public class Enemy extends FlyingObject implements Award{ public static int alive=0; public static int dying=1; public static int dead=2; protected int lifeState=alive; public Enemy(int x, int y, int width, int height) { super(x, y, width, height); } public boolean hit(FlyingObject flyingObjects){ boolean result=false; int x_low=flyingObjects.x-width; int x_up=flyingObjects.x+flyingObjects.width; int y_low=flyingObjects.y-height; int y_up=flyingObjects.y+flyingObjects.height; if(x>x_low&&x<x_up&&y<y_up&&y>y_low){ result=true; } return result; } public boolean impact(Hero hero){ boolean result=false; int x_low=hero.x-width-hero.width/2; int x_up=hero.x+hero.width/2; int y_low=hero.y-height-hero.height/2; int y_up=hero.y+hero.height/2; if(x>x_low&&x<x_up&&y<y_up&&y>y_low){ result=true; } return result; } @Override public int getAward() { return 0; } public void die(){ lifeState=dying; } }
时间: 2023-12-02 11:02:35 浏览: 26
这段代码实现了一个敌人类Enemy,其中包括以下方法:
- 构造方法:生成一个敌人对象,并设置其位置和大小
- hit方法:判断敌人是否被指定的飞行物体击中
- impact方法:判断敌人是否与指定的英雄角色发生碰撞
- getAward方法:获取敌人奖励类型,这里返回0
- die方法:敌人死亡,将生命状态设为dying
该类实现了Award接口,表明敌人可能会掉落奖励道具。
同时,该类继承了FlyingObject类,说明敌人也是一种飞行物体。
这段代码中的注释较少,以下是带中文注释的代码:
```
package Shoot;
import java.awt.Graphics;
@SuppressWarnings("unused")
public class Enemy extends FlyingObject implements Award{
public static int alive=0; // 敌人的三种生命状态
public static int dying=1;
public static int dead=2;
protected int lifeState=alive; // 敌人生命状态,默认为alive
public Enemy(int x, int y, int width, int height) {
super(x, y, width, height); // 构造方法,生成一个敌人对象,并设置其位置和大小
}
public boolean hit(FlyingObject flyingObjects){
boolean result=false;
int x_low=flyingObjects.x-width;
int x_up=flyingObjects.x+flyingObjects.width;
int y_low=flyingObjects.y-height;
int y_up=flyingObjects.y+flyingObjects.height;
if(x>x_low&&x<x_up&&y<y_up&&y>y_low){
result=true; // 判断敌人是否被指定的飞行物体击中
}
return result;
}
public boolean impact(Hero hero){
boolean result=false;
int x_low=hero.x-width-hero.width/2;
int x_up=hero.x+hero.width/2;
int y_low=hero.y-height-hero.height/2;
int y_up=hero.y+hero.height/2;
if(x>x_low&&x<x_up&&y<y_up&&y>y_low){
result=true; // 判断敌人是否与指定的英雄角色发生碰撞
}
return result;
}
@Override
public int getAward() {
return 0; // 获取敌人奖励类型,这里返回0
}
public void die(){
lifeState=dying; // 敌人死亡,将生命状态设为dying
}
}
```
相关推荐
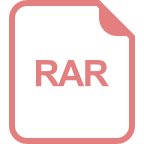
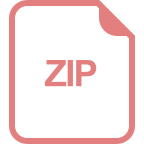














