torch.broadcast_tensors
时间: 2023-06-05 22:06:44 浏览: 214
torch.broadcast_tensors是PyTorch中的一个函数,用于将输入张量广播到具有相同形状的目标形状。广播的过程包括对输入张量的形状进行扩展,使其在另一张量中的维度上具有相同的大小。这个函数可以用来在深度学习的计算过程中进行数据的扩展,以满足某些计算所需的输入张量形状。
相关问题
torch.broadcast_tensors函数讲解
torch.broadcast_tensors函数是PyTorch中用于将张量进行广播运算的函数。在机器学习中,广播运算主要用于将数据进行扩展以便进行计算。比如,计算两个形状不同的张量的和,需要将其中一个张量进行扩展至和另一个张量的形状相同,才能进行计算。
在PyTorch中,torch.broadcast_tensors函数可以将输入的多个张量进行扩展,使它们的形状相同,然后返回扩展后的张量组成的元组。该函数的输入可以是任意数量的张量(至少一个),并且它们的形状必须能够满足广播规则(即能够通过扩展变为相同形状)。
该函数的实现主要依赖于numpy中的broadcast_arrays函数,但要求其输入和输出都是PyTorch张量,因此其返回值是由PyTorch张量组成的元组。该函数返回的所有张量都是新的对象,即它们的数据不共享内存空间。这是为了避免在广播过程中修改原始张量的数据。
例如,以下是使用torch.broadcast_tensors函数将两个张量进行扩展的示例:
```
import torch
x = torch.tensor([1, 2, 3]) # shape: (3,)
y = torch.tensor([[4], [5]]) # shape: (2, 1)
x, y = torch.broadcast_tensors(x, y)
print(x)
print(y)
```
输出结果为:
```
tensor([[1, 2, 3],
[1, 2, 3]])
tensor([[4, 4, 4],
[5, 5, 5]])
```
在该示例中,第一个张量x的形状为(3,),第二个张量y的形状为(2, 1)。经过广播计算后,它们的形状都变为了(2, 3),并且将分别被存储在新的张量对象x和y中。这样就可以对两个形状不同的张量进行计算了。
总之,torch.broadcast_tensors函数是实现PyTorch张量广播计算的重要工具,它可以将多个张量进行扩展,使它们的形状相同并满足广播规则,从而便于进行计算。
torch.nn.functional.mse_loss 的代码
以下是 `torch.nn.functional.mse_loss` 的代码实现:
```python
def mse_loss(input, target, size_average=None, reduce=None, reduction='mean'):
if not (target.size() == input.size()):
warnings.warn("Using a target size ({}) that is different to the input size ({}). "
"This will likely lead to incorrect results due to broadcasting. "
"Please ensure they have the same size.".format(target.size(), input.size()),
stacklevel=2)
if size_average is not None or reduce is not None:
reduction = _Reduction.legacy_get_string(size_average, reduce)
if target.requires_grad:
ret = (input - target) ** 2
if reduction != 'none':
ret = torch.mean(ret) if reduction == 'mean' else torch.sum(ret)
else:
expanded_input, expanded_target = torch.broadcast_tensors(input, target)
ret = torch._C._nn.mse_loss(expanded_input, expanded_target, _Reduction.get_enum(reduction))
return ret
```
该函数计算输入 `input` 和目标 `target` 之间的均方误差(MSE),返回值为标量张量。可选参数 `size_average` 和 `reduce` 被弃用,应使用 `reduction` 参数指定归约方式。参数说明如下:
- `input`:输入张量。
- `target`:目标张量,与输入张量形状相同。
- `size_average`:已弃用。
- `reduce`:已弃用。
- `reduction`:指定用于计算输出张量的归约方式,可选值为 `'none'`、`'mean'` 和 `'sum'`,默认为 `'mean'`。
当 `target.requires_grad=True` 时,计算 `input` 与 `target` 之间的 MSE,并根据 `reduction` 的值进行归约;否则,将 `input` 和 `target` 扩展为相同的形状,再调用 C++ 实现的 `mse_loss` 计算 MSE,并根据 `reduction` 的值进行归约。需要注意的是,如果 `target` 与 `input` 形状不同,该函数会发出警告。
阅读全文
相关推荐
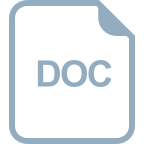
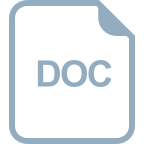
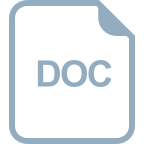



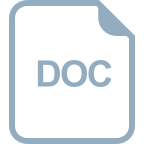
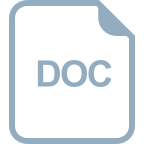
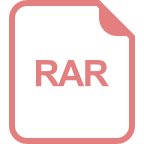
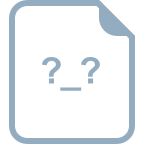
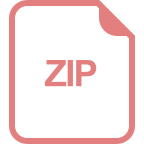
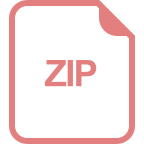