python使用signal对某个响应http请求的方法实现超时监控,(要求使用注解的方式),如果超时需返回自定义的信息但不要通过抛异常的方式,怎么做呢
时间: 2024-03-06 18:48:04 浏览: 14
可以使用Python的`signal`模块来实现超时监控。具体步骤如下:
1. 导入`signal`模块和`functools`模块。
2. 定义一个装饰器函数`timeout`,该函数接受一个超时时间参数`seconds`,并返回一个装饰器函数`decorator`。
3. 在`decorator`函数中,定义一个内部函数`handler`,该函数接受两个参数`signum`和`frame`,并在被调用时抛出`TimeoutError`异常。
4. 在`decorator`函数中,使用`signal.signal`方法将`SIGALRM`信号与`handler`函数进行绑定。
5. 在`decorator`函数中,使用`functools.wraps`方法将原函数的名称、注释等信息复制到包装函数中。
6. 在`decorator`函数中,使用`time`模块的`time`方法记录函数执行开始时间。
7. 在`decorator`函数中,使用`signal.alarm`方法设置超时时间。
8. 在`decorator`函数中,使用`try-except`语句块执行原函数,并在超时时捕获`TimeoutError`异常。
9. 在`decorator`函数中,使用`signal.alarm(0)`方法取消超时时间。
10. 在`decorator`函数中,如果超时,则返回自定义的信息;否则,返回原函数的返回值。
下面是一个示例代码:
```python
import signal
import functools
import time
class TimeoutError(Exception):
pass
def timeout(seconds):
def decorator(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
def handler(signum, frame):
raise TimeoutError("Function execution time out")
signal.signal(signal.SIGALRM, handler)
signal.alarm(seconds)
start_time = time.time()
try:
result = func(*args, **kwargs)
except TimeoutError:
return "Function execution time out"
finally:
signal.alarm(0)
end_time = time.time()
print("Function execution time: %.2f seconds" % (end_time - start_time))
return result
return wrapper
return decorator
```
使用该装饰器函数对某个响应HTTP请求的方法进行装饰即可实现超时监控。例如:
```python
@timeout(5)
def handle_request(request):
# 处理请求
time.sleep(10)
return "OK"
```
上述代码表示,对`handle_request`方法进行超时监控,超时时间为5秒。如果`handle_request`方法在5秒内执行完毕,则返回"OK";否则,返回"Function execution time out"。同时,如果超时,不会抛出异常,而是直接返回自定义的信息。
相关推荐
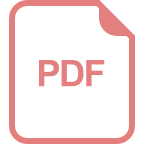
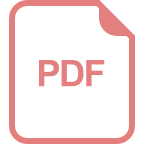
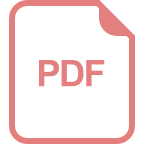















