爬取京东商品参数的python代码
时间: 2023-12-29 09:04:13 浏览: 30
以下是一个简单的使用 Python 和 Beautiful Soup 库来爬取京东商品参数的示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://item.jd.com/123456.html' # 将 123456 替换成你要爬取的商品编号
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 获取商品名称
name = soup.find('div', {'class': 'sku-name'}).text.strip()
print('商品名称:', name)
# 获取商品参数
params = soup.find('div', {'class': 'p-parameter'}).find_all('li')
print('商品参数:')
for param in params:
print(param.text.strip())
```
需要注意的是,需要将 `url` 中的 123456 替换成你要爬取的商品编号。此外,不同商品的页面结构可能不同,因此需要根据实际情况调整代码。
相关问题
爬取京东网站数据 python代码
以下是爬取京东商品数据的 Python 代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头信息,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Edge/16.16299'
}
# 定义爬取函数
def get_jd_items(keyword):
# 构造请求 URL
url = 'https://search.jd.com/Search'
params = {
'keyword': keyword,
'enc': 'utf-8',
'wq': keyword,
'page': '1'
}
# 发送 GET 请求获取页面内容
response = requests.get(url, params=params, headers=headers)
# 使用 BeautifulSoup 解析页面内容
soup = BeautifulSoup(response.text, 'html.parser')
# 获取商品列表
items = soup.select('#J_goodsList > ul > li')
# 遍历商品列表,提取商品信息
for item in items:
# 商品名称
name = item.select('.p-name > a > em')[0].text
# 商品价格
price = item.select('.p-price > strong > i')[0].text
# 商品链接
link = item.select('.p-name > a')[0]['href']
# 商品图片链接
image = item.select('.p-img > a > img')[0]['src']
# 打印商品信息
print('名称:', name)
print('价格:', price)
print('链接:', link)
print('图片:', image)
print('-' * 50)
# 调用爬取函数
get_jd_items('手机')
```
上述代码会爬取京东搜索页面中第一页的手机商品信息,可以根据需要修改关键词和页码等参数。需要注意的是,网站的反爬机制可能会对请求进行限制,可以通过设置请求头信息等方式进行规避。
python爬取京东商品评论
好的,您可以使用 Python 中的 requests 和 BeautifulSoup 库来爬取京东商品评论。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://sclub.jd.com/comment/productPageComments.action?productId=100000177760&score=0&sortType=5&page=0&pageSize=10'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
comments = soup.select('div.comment-column J-comment-column > div.comment-con > div > div.comment-content > p')
for comment in comments:
print(comment.text.strip())
```
注意:需要替换 URL 中的 `productId` 参数为你想要爬取评论的商品 ID。另外,在爬取京东评论时需要注意反爬策略,建议添加适当的延迟和 User-Agent 头信息。
相关推荐
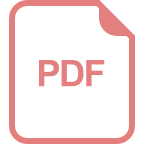
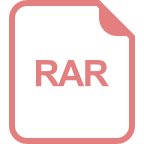












