#include<iostream> #include<vector> #include<string> using namespace std; class Employee { public: virtual void display() = 0; virtual double salary() = 0; Employee(int a=0, string b=0) :id(a), name(b) { } ~Employee() { } protected: int id; string name; }; class Salesman:public Employee { public: Salesman(int a1 ,string b1 ,double a = 0,double c=0 ,int b = 0 ) :basesalary(a), amountofsales(b),comissionRate(c),Employee(a1,b1) { } ~Salesman() { } virtual double salary() { return (basesalary + amountofsales * comissionRate); } virtual void display() { cout << id << "-" << name << "-" << basesalary << "-" << amountofsales << "salary=" << salary() << endl; } protected: double basesalary; int amountofsales; double comissionRate; }; class Technician :public Employee { public: Technician(int a1,string b1,double a,int b,double c):basesalary(a),workhours(b),paymenthour(c),Employee(a1,b1) { } ~Technician() { } virtual double salary() { return (basesalary + workhours * paymenthour); } virtual void dispaly() { cout << id << "-" << name << "-" << basesalary << "-" << workhours <<"-" << paymenthour<<"-" << salary() << endl; } protected: double basesalary; int workhours; double paymenthour; }; int main() { vector<Employee*>e(4); e[0] = new Salesman(1001, "Dal", 1000.0, 0.04, 10000); e[1] = new Salesman(1002, "Viv", 1100.0, 0.06, 7000); e[2] = new Technician(1003, "Chirs", 1100.0, 15, 60.0); e[3]= new Technician(1004, "Chi", 1100.0, 18, 40.0); for (int i = 0;i < e.size();i++) { e[i]-> display(); } for (int i = 0;i < e.size();i++) { delete e[i]; } }
时间: 2024-04-27 20:25:00 浏览: 11
这是一个基于多态的简单的员工管理系统的代码。其中有一个抽象基类 Employee,它有两个纯虚函数 display 和 salary,分别用于显示员工信息和计算员工薪水。派生类 Salesman 和 Technician 继承自 Employee 类,并实现了纯虚函数。在 main 函数中,使用了一个指向 Employee 类的指针数组来管理员工,通过向这个数组添加不同的派生类对象实现了多态性。最后在程序结束前,释放了动态分配的内存。
相关问题
#include <iostream> #include <fstream> #include <vector> #include <string> using namespace std; //
这是一段C++程序的头文件部分,包含了一些标准库的头文件,具体介绍如下:
1. iostream:提供了输入输出流的基本功能,如cin、cout等。
2. fstream:提供了文件操作相关的功能,如读写文件等。
3. vector:提供了可变大小数组的功能,可以方便地进行动态管理。
4. string:提供了字符串相关的功能,如字符串的拼接、截取等。
其中,using namespace std; 表示使用标准命名空间,可以简化程序中的代码。
解释代码# include < iostream > # include < string > # include < vector > # include < iomanip > using namespace std ;
这段代码是 C++ 中的预处理指令,用于引入头文件并进行命名空间声明。
- `#include <iostream>` 引入输入输出流库,用于控制台输入输出。
- `#include <string>` 引入字符串库,用于处理字符串操作。
- `#include <vector>` 引入向量库,用于动态数组操作。
- `#include <iomanip>` 引入输入输出流格式化库,用于格式化控制台输出。
`using namespace std;` 是命名空间声明,表示使用标准命名空间(std)中的所有函数和类,避免了每次使用时都要写 std:: 的麻烦。
相关推荐
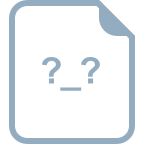













