使用【c语言】写【写出一棵赫夫曼树,并根据其求赫夫曼编码的算法,用户键盘敲击若干个整数作为代编码字符的权值,程序构造赫夫曼树,并输出赫夫曼编码】的代码
时间: 2024-12-20 10:17:36 浏览: 25
在 C 语言中,实现赫夫曼树并生成对应的编码通常涉及到数据结构、优先队列以及递归等概念。以下是简化的步骤和一个基本的示例代码框架:
首先,我们需要创建一个结构体来存储字符及其权值,并定义一个节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
char data;
int weight;
struct Node* left;
struct Node* right;
} Node;
typedef struct PriorityQueue {
Node** queue;
int size;
int capacity;
} PriorityQueue;
```
接下来,定义一些函数:
1. 初始化优先队列
2. 插入节点到队列
3. 删除最小权重节点
4. 构建赫夫曼树
5. 打印赫夫曼编码
下面是一个简化版的赫夫曼树构建和编码生成的算法:
```c
// 建立优先队列
void initQueue(PriorityQueue* pq) {
pq->queue = (Node**)malloc(sizeof(Node*) * pq->capacity);
pq->size = 0;
}
// 插入节点
void insert(PriorityQueue* pq, Node* node) {
if (pq->size == pq->capacity) {
pq->capacity *= 2;
pq->queue = realloc(pq->queue, sizeof(Node*) * pq->capacity);
}
pq->queue[pq->size++] = node;
}
// 删除最小节点
Node* extractMin(PriorityQueue* pq) {
if (pq->size == 0)
return NULL;
Node* minNode = pq- 1];
pq->size--;
pq->queue[pq->size] = NULL;
return minNode;
}
// 构建赫夫曼树
Node* buildHuffmanTree(PriorityQueue* pq) {
// ... (这里需要递归地合并最小节点直到只剩下一个节点)
}
// 求解赫夫曼编码
void huffmanCode(Node* root, char* code, char lastCodeChar) {
if (root == NULL) return;
if (root->left == NULL && root->right == NULL) { // 赫夫曼树叶节点
code[root->data] = lastCodeChar + 1;
printf("%c ", lastCodeChar + 1); // 输出编码
} else {
huffmanCode(root->left, code, '0');
huffmanCode(root->right, code, '1');
}
}
int main() {
PriorityQueue pq;
initQueue(&pq);
// 用户输入字符和权值,插入队列
// ...
// 构建赫夫曼树
Node* root = buildHuffmanTree(&pq);
// 获取每个字符的赫夫曼编码
char code[256] = {0}; // 预备存放编码
huffmanCode(root, code, '\0');
// 输出赫夫曼编码
for (int i = 0; i < 256; i++) {
printf("Character %c has Huffman Code %d\n", i, code[i]);
}
free(pq.queue);
return 0;
}
```
注意,上述代码是一个简化版本,实际实现中还需要处理用户输入、优先队列的具体操作(如维护堆性质),以及合并节点的递归过程。完整的代码可能超过此处的范围,但这个框架应该能帮助你开始编写。
阅读全文
相关推荐
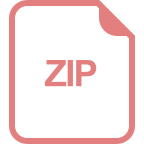
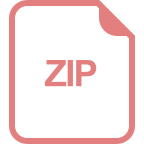
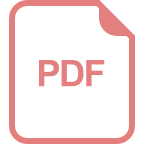
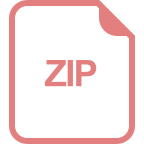
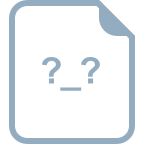
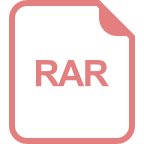
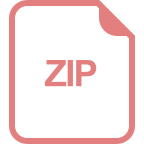
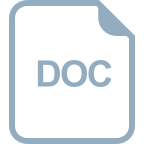







