请用Verilog写出上述要求
时间: 2024-09-19 17:01:21 浏览: 33
在Verilog硬件描述语言中,实现一个按32点窗口长度、31点重叠进行的16位浮点复数输入的短时傅里叶变换(STFT),我们需要使用`rams`(随机存取存储器)、`for`循环和`if`条件语句。由于Verilog更偏向于描述硬件逻辑,所以直接操作数据块的方式会比较直观。
```verilog
module stft_module(
input [15:0] real_input [0 : N-1], // 输入16位实部和虚部组成的复数数组
output reg [15:0] real_output [0 : M-1],
output reg [15:0] imag_output [0 : M-1]
);
// 窗口大小和重叠
parameter W = 32;
parameter O = 31;
// 计算有效帧数和步长
localparam num_frames = ceil((N - W) / (W - O));
localparam step = W - O;
// RAMs for storing the overlapping windows
reg [15:0] ram_real [0 : W-1];
reg [15:0] ram_imag [0 : W-1];
// 对于每一个窗口
for (integer i = 0; i < num_frames; i++) begin
integer k = i * step; // 当前窗口开始位置
// 读取输入并存储到RAM中
for (integer j = 0; j < W; j++) begin
if (j < N) begin
ram_real[j] = real_input[k+j];
ram_imag[j] = ...; // 这里假设虚部也存在同样的RAM中,并复制过来
end else begin
ram_real[j] = 0; // 如果超过输入范围,设置为0
ram_imag[j] = 0;
end
end
// 对RAM内的窗口做FFT
real_output[i*step:i*step+W-1] = fft(ram_real);
imag_output[i*step:i*step+W-1] = fft(ram_imag); // 假设fft函数返回的是实部和虚部的数组
end
endmodule
// 注意:在实际设计中,你需要定义一个外部FFT模块(如your_fft_module)来计算窗口的FFT
function [15:0] fft([15:0] real_data [0 : W-1]);
// 假设your_fft_module能完成16位输入的复数FFT,并返回两个16位数组
your_fft_module(your_fft_params, real_data, {real_output, imag_output});
endfunction
阅读全文
相关推荐
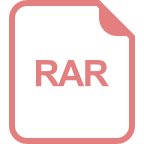
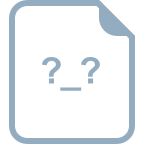
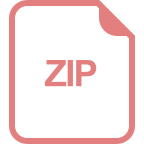















