使用RSA算法加密字符串
时间: 2024-06-09 11:01:32 浏览: 6
使用RSA算法加密字符串的步骤如下:
1. 生成RSA密钥对,包括公钥和私钥。
2. 将要加密的字符串转换为字节数组。
3. 使用公钥对字节数组进行加密。
4. 将加密后的字节数组转换为Base64编码的字符串。
以下是Python中使用pycryptodome库实现RSA加密字符串的示例代码:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_v1_5
import base64
# 生成RSA密钥对
key = RSA.generate(2048)
# 获取公钥和私钥
private_key = key.export_key()
public_key = key.publickey().export_key()
# 将要加密的字符串转换为字节数组
message = b'This is a secret message.'
# 使用公钥对字节数组进行加密
cipher = PKCS1_v1_5.new(RSA.import_key(public_key))
ciphertext = cipher.encrypt(message)
# 将加密后的字节数组转换为Base64编码的字符串
encrypted_message = base64.b64encode(ciphertext).decode('utf-8')
print('加密后的字符串:', encrypted_message)
```
相关问题
使用RSA算法加密字符串C++
使用RSA算法加密字符串的步骤如下:
1.生成RSA密钥对,包括公钥和私钥。可以使用openssl库中的RSA_generate_key函数生成密钥对。
2.使用公钥对明文进行加密。可以使用openssl库中的RSA_public_encrypt函数进行加密。
3.使用私钥对密文进行解密。可以使用openssl库中的RSA_private_decrypt函数进行解密。
下面是一个使用openssl库实现RSA加密的C++代码示例:
```c++
#include <openssl/rsa.h>
#include <openssl/pem.h>
int main()
{
// 生成RSA密钥对
RSA *keypair = RSA_generate_key(2048, RSA_F4, NULL, NULL);
if (keypair == NULL) {
printf("Failed to generate RSA key pair\n");
return -1;
}
// 明文
const char *plaintext = "Hello, world!";
// 计算加密后的密文长度
int ciphertext_len = RSA_size(keypair);
unsigned char *ciphertext = new unsigned char[ciphertext_len];
// 使用公钥加密明文
int len = RSA_public_encrypt(strlen(plaintext) + 1, (unsigned char *)plaintext, ciphertext, keypair, RSA_PKCS1_PADDING);
if (len == -1) {
printf("Failed to encrypt plaintext\n");
return -1;
}
// 计算解密后的明文长度
int plaintext_len = RSA_size(keypair);
unsigned char *decrypted = new unsigned char[plaintext_len];
// 使用私钥解密密文
len = RSA_private_decrypt(ciphertext_len, ciphertext, decrypted, keypair, RSA_PKCS1_PADDING);
if (len == -1) {
printf("Failed to decrypt ciphertext\n");
return -1;
}
// 输出解密后的明文
printf("Decrypted plaintext: %s\n", decrypted);
// 释放内存
RSA_free(keypair);
delete[] ciphertext;
delete[] decrypted;
return 0;
}
```
java使用RSA加密字符串
Java中使用RSA加密字符串的步骤如下:
1. 生成RSA密钥对
```java
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
```
2. 获取公钥和私钥
```java
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
```
3. 使用公钥加密字符串
```java
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
```
4. 使用私钥解密字符串
```java
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
String decryptedText = new String(decryptedBytes);
```
完整代码示例:
```java
import java.security.*;
import javax.crypto.*;
public class RSAEncryptionExample {
public static void main(String[] args) throws Exception {
String plainText = "Hello, world!";
// 生成RSA密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
// 获取公钥和私钥
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 使用公钥加密字符串
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
// 使用私钥解密字符串
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
String decryptedText = new String(decryptedBytes);
System.out.println("Plain Text: " + plainText);
System.out.println("Encrypted Text: " + new String(encryptedBytes));
System.out.println("Decrypted Text: " + decryptedText);
}
}
```
注意:RSA加密算法是一种非常耗时的加密算法,因此在加密大量数据时会影响性能。建议使用对称加密算法来加密大量数据。
相关推荐
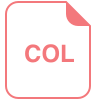
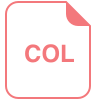
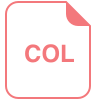
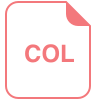
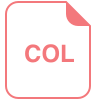







