torch.nn.sequential apply
时间: 2023-11-07 08:01:47 浏览: 180
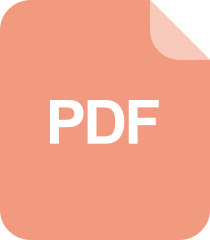
对Pytorch中nn.ModuleList 和 nn.Sequential详解
torch.nn.Sequential是一个容器,用于按顺序组织神经网络的模块。它的apply方法可以应用一个函数(如init_weights)到Sequential容器中的每个模块。具体来说,在apply内部,它会遍历Sequential的子模块并应用给定的函数,然后对自身应用该函数。在上面的示例中,当调用net.apply(init_weights)时,init_weights函数被应用到Sequential容器中的每个nn.Linear模块。
阅读全文
相关推荐















