用C++写一个程序实例,去除txt文件中的中文或英文的停用词,其中停用词表为a,an,the,of,的,一,了
时间: 2023-10-08 15:05:25 浏览: 87
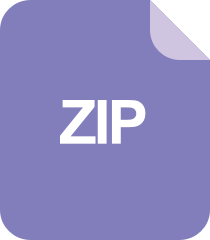
文本分词常用中文停用词表
由于不知道停用词表的具体格式,因此以下程序实例只针对单行文本进行停用词去除。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 停用词表
char stopwords[6][4] = {"a", "an", "the", "of", "的", "一"};
// 判断一个单词是否为停用词
int is_stopword(char* word) {
int i;
for (i = 0; i < 6; i++) {
if (strcmp(word, stopwords[i]) == 0) {
return 1;
}
}
return 0;
}
int main() {
FILE* fp = fopen("test.txt", "r"); // 打开文件
char line[1024]; // 定义存储一行文本的字符数组
char* word; // 定义指向单词的指针
char* delim = " ,.!?\n"; // 定义分隔符
while (fgets(line, 1024, fp) != NULL) { // 逐行读取文本
word = strtok(line, delim); // 以分隔符为界限,切割单词
while (word != NULL) {
if (!is_stopword(word)) { // 如果不是停用词,则输出
printf("%s ", word);
}
word = strtok(NULL, delim); // 继续切割
}
}
fclose(fp); // 关闭文件
return 0;
}
```
需要注意的是,在判断单词是否为停用词的函数中,我们使用了 `strcmp` 函数进行比较。另外,在读取文本的过程中,我们采用了 `fgets` 函数,以避免一次读取过多字符导致内存溢出的问题。
阅读全文
相关推荐
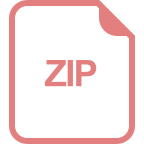
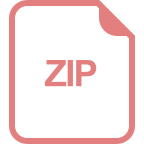















