用C++写一个程序实例,去除例子txt文件中的中文或英文的停用词,其中停用词表
时间: 2024-05-12 22:19:56 浏览: 162
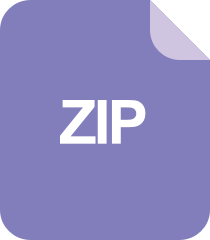
文本分词常用中文停用词表
可以自行选择或下载。以下是一个简单例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 50
#define MAX_STOP_WORDS 1000
/* 读取停用词表 */
int read_stop_words(char stop_words[][MAX_WORD_LEN], const char* filename) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open stop words file: %s\n", filename);
return 0;
}
int num_words = 0;
while (fgets(stop_words[num_words], MAX_WORD_LEN, fp) != NULL) {
// 去除换行符
stop_words[num_words][strcspn(stop_words[num_words], "\n")] = '\0';
num_words++;
if (num_words >= MAX_STOP_WORDS) {
printf("Stop words table is full (%d words)\n", MAX_STOP_WORDS);
break;
}
}
fclose(fp);
return num_words;
}
/* 判断是否为停用词 */
int is_stop_word(const char* word, char stop_words[][MAX_WORD_LEN], int num_stop_words) {
for (int i = 0; i < num_stop_words; i++) {
if (strcmp(word, stop_words[i]) == 0) {
return 1;
}
}
return 0;
}
/* 去除停用词 */
void remove_stop_words(const char* input_filename, const char* output_filename, char stop_words[][MAX_WORD_LEN], int num_stop_words) {
FILE* fp_in = fopen(input_filename, "r");
if (fp_in == NULL) {
printf("Failed to open input file: %s\n", input_filename);
return;
}
FILE* fp_out = fopen(output_filename, "w");
if (fp_out == NULL) {
printf("Failed to open output file: %s\n", output_filename);
fclose(fp_in);
return;
}
char word[MAX_WORD_LEN];
while (fscanf(fp_in, "%s", word) == 1) {
if (!is_stop_word(word, stop_words, num_stop_words)) {
fprintf(fp_out, "%s ", word);
}
}
fclose(fp_in);
fclose(fp_out);
}
int main(void) {
char stop_words[MAX_STOP_WORDS][MAX_WORD_LEN];
int num_stop_words = read_stop_words(stop_words, "stop_words.txt");
if (num_stop_words == 0) {
return 1;
}
remove_stop_words("example.txt", "example_no_stop_words.txt", stop_words, num_stop_words);
return 0;
}
```
其中,`read_stop_words`函数用于读取停用词表,`is_stop_word`函数用于判断单词是否为停用词,`remove_stop_words`函数用于去除停用词。在`main`函数中,首先读取停用词表,然后调用`remove_stop_words`函数去除停用词,最后生成一个新的文件。这个例子假设输入文件中的单词都是以空格分隔的,如果需要处理其他分隔符,可以修改`remove_stop_words`函数。
阅读全文
相关推荐
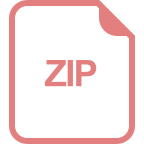
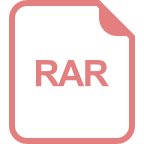















