ssh_get_error 在c语言中错误信息打印
时间: 2023-04-01 15:01:22 浏览: 183
ssh_get_error 函数可以用于获取 SSH 库中的错误信息,它返回一个错误代码,可以通过 ssh_err() 函数将其转换为错误信息字符串。例如:
int rc = ssh_get_error(session);
char *errmsg = ssh_err(rc);
这样就可以得到一个字符串 errmsg,其中包含了 SSH 库中的错误信息。需要注意的是,如果 rc 的值为 SSH_OK,则表示没有错误发生。
相关问题
C语言ssh_getaddrinfo函数详解
`ssh_getaddrinfo`并不是C语言的标准库函数,它看起来可能是某个SSH(Secure Shell)库中的特定功能,比如OpenSSH或者某些SSH客户端库提供的API。这个函数通常用于解析SSH连接目标主机的地址信息,例如从给定的主机名或IP地址获取地址描述符(address info),以便后续建立网络连接。
在实际使用中,这个函数可能会接收一个字符串作为输入(如`hostname`或`service`),然后返回一个指向`struct addrinfo`链表的指针,其中包含了关于如何连接到该主机的各种信息,如协议、端口等。`struct addrinfo`定义了包含这些信息的数据结构,包括family(协议族)、socktype(套接字类型)、protocol(协议级别)和socket地址。
然而,由于这不是标准C库的一部分,具体的函数原型、用法和错误处理方式会依赖于所使用的SSH库的文档。例如,在libssh2这样的库中,你可能需要像下面这样调用:
```c
struct addrinfo* res = NULL;
int error = ssh_getaddrinfo(socket, "hostname", &hints, &res);
if (error) {
// 处理错误
}
// 使用res进行连接...
freeaddrinfo(res); // 当不再需要时释放内存
```
c语言连接ssh服务器
要连接 SSH 服务器,需要使用 SSH 客户端,例如 OpenSSH。在 C 语言中,可以使用 libssh 库来实现 SSH 连接和通信。
以下是一个简单的 C 语言程序,使用 libssh 库连接到 SSH 服务器并执行命令:
```c
#include <stdio.h>
#include <stdlib.h>
#include <libssh/libssh.h>
int main() {
ssh_session my_ssh_session;
int rc;
my_ssh_session = ssh_new();
if (my_ssh_session == NULL) {
fprintf(stderr, "Error creating SSH session\n");
exit(1);
}
ssh_options_set(my_ssh_session, SSH_OPTIONS_HOST, "example.com");
ssh_options_set(my_ssh_session, SSH_OPTIONS_USER, "username");
rc = ssh_connect(my_ssh_session);
if (rc != SSH_OK) {
fprintf(stderr, "Error connecting to SSH server: %s\n", ssh_get_error(my_ssh_session));
ssh_free(my_ssh_session);
exit(1);
}
rc = ssh_userauth_password(my_ssh_session, NULL, "password");
if (rc != SSH_AUTH_SUCCESS) {
fprintf(stderr, "Error authenticating with SSH server: %s\n", ssh_get_error(my_ssh_session));
ssh_disconnect(my_ssh_session);
ssh_free(my_ssh_session);
exit(1);
}
ssh_channel channel;
int nbytes;
char buffer[256];
channel = ssh_channel_new(my_ssh_session);
if (channel == NULL) {
fprintf(stderr, "Error creating SSH channel\n");
ssh_disconnect(my_ssh_session);
ssh_free(my_ssh_session);
exit(1);
}
rc = ssh_channel_open_session(channel);
if (rc != SSH_OK) {
fprintf(stderr, "Error opening SSH channel: %s\n", ssh_get_error(my_ssh_session));
ssh_channel_free(channel);
ssh_disconnect(my_ssh_session);
ssh_free(my_ssh_session);
exit(1);
}
rc = ssh_channel_request_exec(channel, "ls -l");
if (rc != SSH_OK) {
fprintf(stderr, "Error executing command on SSH channel: %s\n", ssh_get_error(my_ssh_session));
ssh_channel_close(channel);
ssh_channel_free(channel);
ssh_disconnect(my_ssh_session);
ssh_free(my_ssh_session);
exit(1);
}
nbytes = ssh_channel_read(channel, buffer, sizeof(buffer), 0);
while (nbytes > 0) {
fwrite(buffer, 1, nbytes, stdout);
nbytes = ssh_channel_read(channel, buffer, sizeof(buffer), 0);
}
ssh_channel_send_eof(channel);
ssh_channel_close(channel);
ssh_channel_free(channel);
ssh_disconnect(my_ssh_session);
ssh_free(my_ssh_session);
return 0;
}
```
在上面的示例中,使用 `ssh_options_set()` 函数设置 SSH 服务器的主机名和用户名,使用 `ssh_connect()` 函数连接到 SSH 服务器,使用 `ssh_userauth_password()` 函数进行密码身份验证。然后使用 `ssh_channel_new()` 函数创建 SSH 通道,使用 `ssh_channel_open_session()` 函数打开会话通道,使用 `ssh_channel_request_exec()` 函数在通道上执行命令,并使用 `ssh_channel_read()` 函数读取命令输出。最后关闭通道和 SSH 会话。
请注意,该示例中使用密码进行身份验证,这并不安全。建议使用 SSH 密钥身份验证来提高安全性。
阅读全文
相关推荐
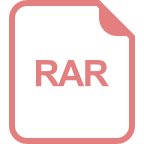
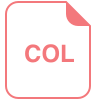
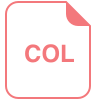
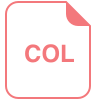
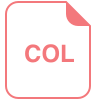








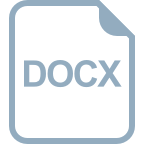