帮我写一段c语言在Linux下基于libssh的sftp登录和下载文件代码
时间: 2024-02-09 18:09:32 浏览: 29
好的,以下是基于libssh的sftp登录和下载文件的C语言代码,适用于Linux系统:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libssh/libssh.h>
#include <libssh/sftp.h>
int sftp_download(ssh_session session, const char *remote_path, const char *local_path) {
sftp_session sftp = sftp_new(session);
if (sftp == NULL) {
fprintf(stderr, "Error: Failed to create SFTP session.\n");
return SSH_ERROR;
}
if (sftp_init(sftp) != SSH_OK) {
fprintf(stderr, "Error: Failed to initialize SFTP session: %s.\n", ssh_get_error(session));
sftp_free(sftp);
return SSH_ERROR;
}
sftp_file remote_file = sftp_open(sftp, remote_path, O_RDONLY, 0);
if (remote_file == NULL) {
fprintf(stderr, "Error: Failed to open remote file: %s.\n", ssh_get_error(session));
sftp_free(sftp);
return SSH_ERROR;
}
FILE *local_file = fopen(local_path, "w");
if (local_file == NULL) {
fprintf(stderr, "Error: Failed to open local file: %s.\n", strerror(errno));
sftp_close(remote_file);
sftp_free(sftp);
return SSH_ERROR;
}
char buffer[1024];
ssize_t bytes_read;
while ((bytes_read = sftp_read(remote_file, buffer, sizeof(buffer))) > 0) {
fwrite(buffer, 1, bytes_read, local_file);
}
if (bytes_read < 0) {
fprintf(stderr, "Error: Failed to read remote file: %s.\n", ssh_get_error(session));
fclose(local_file);
sftp_close(remote_file);
sftp_free(sftp);
return SSH_ERROR;
}
fclose(local_file);
sftp_close(remote_file);
sftp_free(sftp);
printf("Downloaded file from %s to %s.\n", remote_path, local_path);
return SSH_OK;
}
int main(int argc, char **argv) {
if (argc != 5) {
fprintf(stderr, "Usage: %s <host> <port> <user> <password>\n", argv[0]);
return EXIT_FAILURE;
}
const char *host = argv[1];
const int port = atoi(argv[2]);
const char *user = argv[3];
const char *password = argv[4];
ssh_session session = ssh_new();
if (session == NULL) {
fprintf(stderr, "Error: Failed to create SSH session.\n");
return EXIT_FAILURE;
}
ssh_options_set(session, SSH_OPTIONS_HOST, host);
ssh_options_set(session, SSH_OPTIONS_PORT, &port);
ssh_options_set(session, SSH_OPTIONS_USER, user);
if (ssh_connect(session) != SSH_OK) {
fprintf(stderr, "Error: Failed to connect: %s.\n", ssh_get_error(session));
ssh_free(session);
return EXIT_FAILURE;
}
if (ssh_userauth_password(session, NULL, password) != SSH_AUTH_SUCCESS) {
fprintf(stderr, "Error: Failed to authenticate: %s.\n", ssh_get_error(session));
ssh_disconnect(session);
ssh_free(session);
return EXIT_FAILURE;
}
const char *remote_path = "/path/to/remote/file";
const char *local_path = "/path/to/local/file";
if (sftp_download(session, remote_path, local_path) != SSH_OK) {
ssh_disconnect(session);
ssh_free(session);
return EXIT_FAILURE;
}
ssh_disconnect(session);
ssh_free(session);
return EXIT_SUCCESS;
}
```
请注意将 `<host>`, `<port>`, `<user>` 和 `<password>` 替换为实际的值,以及将 `"/path/to/remote/file"` 和 `"/path/to/local/file"` 替换为实际的远程和本地文件路径。
相关推荐
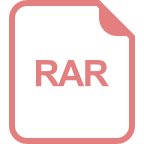














