键盘输入任意的三个字符串,实现文本的查找替换功能。如: String source="This is Java program, Java is good ......."; String find="Java"; String dest="Java language"; 要求: (1)将source串中的第一个字符串 find,用字符串dest替换。 (2)仅替换最后的一个串find (3)将source中的所有 find串替换为字符串 dest。 (4)统计 source串中共有多少个单词。 打出完整代码,从创建包开始
时间: 2024-02-24 14:59:36 浏览: 15
好的,以下是完整的 Java 代码:
```java
package com.example;
import java.util.Scanner;
public class TextReplace {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入源字符串:");
String source = scanner.nextLine();
System.out.print("请输入要查找的字符串:");
String find = scanner.nextLine();
System.out.print("请输入要替换为的字符串:");
String dest = scanner.nextLine();
// 替换第一个字符串
String replaced1 = source.replaceFirst(find, dest);
System.out.println("替换第一个字符串后的结果:" + replaced1);
// 替换最后一个字符串
String replaced2 = source.replace(find, dest);
int lastIndex = replaced2.lastIndexOf(dest);
int lastFindIndex = replaced2.lastIndexOf(find);
if (lastFindIndex >= 0 && lastIndex >= 0 && lastIndex > lastFindIndex) {
replaced2 = replaced2.substring(0, lastFindIndex) + dest +
replaced2.substring(lastFindIndex + find.length());
}
System.out.println("替换最后一个字符串后的结果:" + replaced2);
// 替换所有字符串
String replaced3 = source.replaceAll(find, dest);
System.out.println("替换所有字符串后的结果:" + replaced3);
// 统计单词数量
int wordCount = source.split("\\W+").length;
System.out.println("单词数量为:" + wordCount);
}
}
```
这个程序会提示用户输入源字符串、要查找的字符串和要替换为的字符串,然后依次执行题目中所要求的四个操作,并输出结果。其中,第一个操作使用了 `String` 类的 `replaceFirst` 方法;第二个操作则使用 `String` 类的 `replace` 方法和 `lastIndexOf` 方法;第三个操作使用 `String` 类的 `replaceAll` 方法;最后一个操作使用 `String` 类的 `split` 方法和正则表达式。
需要注意的一点是,如果替换的字符串中包含要查找的字符串,那么在进行最后一个操作时,可能会出现统计单词数量不准确的情况。
阅读全文
相关推荐
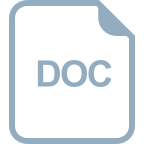
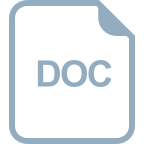
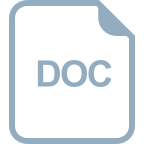







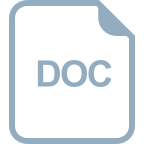
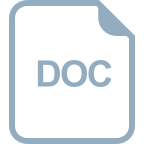
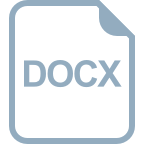
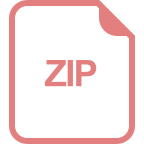
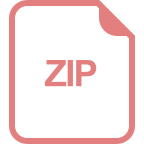
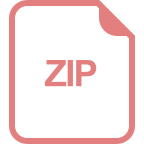
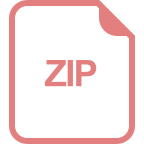